How to Solve SVM Assignments Using MATLAB
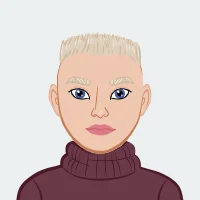
Support Vector Machines (SVM) are a cornerstone of modern machine learning, especially in classification problems. If you're a student working on SVM assignments using MATLAB, you might feel overwhelmed by the mathematical concepts and programming tasks involved. This blog will guide you through a systematic approach to solve your SVM assignments using MATLAB, focusing on plotting data points, calculating hyperplanes, and finding maximum margins. Let's dive in!
Understanding the Assignment Requirements
Before you begin coding, it's crucial to thoroughly read and understand the assignment requirements. Typically, an SVM assignment may ask you to:
- Plot data points for two classes (positive and negative).
- Select support vectors.
- Calculate and plot the hyperplane.
- Calculate and plot the margin planes.
- Compute the maximum margin value.
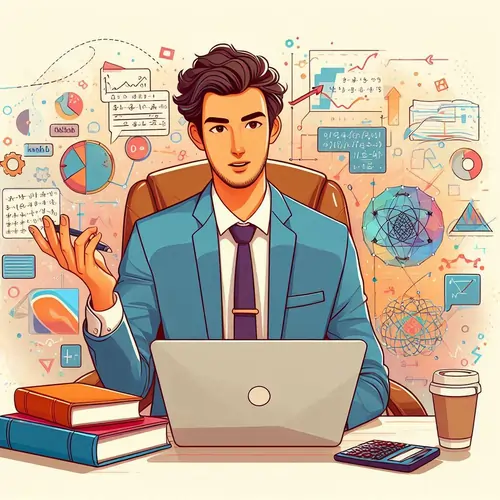
Having a clear understanding of these requirements will help you plan your approach and avoid common pitfalls.
Setting Up Your Environment
Ensure that MATLAB is installed and running on your computer. MATLAB is a powerful tool for matrix computations and data visualization, making it ideal for machine learning tasks like SVM. Open MATLAB and create a new script file where you will write your code.
Loading and Plotting Data
The first step is to load and plot your data points. Let's assume you have two sets of 2D data points representing the negative and positive classes. You can use the following MATLAB code to define and plot these data points:
% Define data points
% Define data points
AA = [9,9; 10,5; 16,8; 14,1; 15,2; 12,8; 12,2; 15,6; 10,12; 13,13];
BB = [-2,3; 1,6; 1,2; 2,4; -3,9; -1,5; -1,1; -5,6; -2,5; -4,3];
% Plot data points
figure;
hold on;
scatter(AA(:,1), AA(:,2), 'r', 'filled'); % Negative class in red
scatter(BB(:,1), BB(:,2), 'g', 'filled'); % Positive class in green
legend('Negative Class', 'Positive Class');
title('2D Data Points for Two Classes');
xlabel('X-axis');
ylabel('Y-axis');
hold off;
This code snippet initializes the data points and plots them on a 2D graph, with red dots representing the negative class and green dots representing the positive class.
Training the SVM
Once your data is plotted, the next step is to train the SVM model. MATLAB provides built-in functions to train SVM models, which simplifies the process significantly. The fitcsvm function is particularly useful for this purpose.
% Combine data sets and create labels
data = [AA; BB];
labels = [-1 * ones(size(AA, 1), 1); ones(size(BB, 1), 1)];
% Train SVM model
SVMModel = fitcsvm(data, labels, 'KernelFunction', 'linear', 'Standardize', true);
% Extract support vectors
supportVectors = SVMModel.SupportVectors;
% Plot support vectors
hold on;
scatter(supportVectors(:,1), supportVectors(:,2), 'ko', 'filled'); % Support vectors in black
In this code, we combine the data sets and create labels for each class (negative class labeled as -1 and positive class labeled as 1). We then train the SVM model using a linear kernel and standardize the data. The support vectors identified by the SVM model are plotted in black.
Calculating and Plotting the Hyperplane
The hyperplane is a key component of the SVM model. It is the decision boundary that separates the classes. The coefficients of the hyperplane can be extracted from the trained SVM model.
% Get hyperplane parameters
w = SVMModel.Beta;
b = SVMModel.Bias;
% Equation of the hyperplane: w1*x1 + w2*x2 + b = 0
% Calculate hyperplane
x = linspace(min(data(:,1)), max(data(:,1)), 100);
y = -(w(1) * x + b) / w(2);
% Plot hyperplane
plot(x, y, 'b-'); % Hyperplane in blue
Here, we extract the hyperplane parameters (coefficients) and calculate the hyperplane equation. We then plot the hyperplane in blue. The linspace function generates a vector of 100 points between the minimum and maximum values of the data, ensuring a smooth line for the hyperplane.
Calculating and Plotting Margin Planes
Margin planes are parallel to the hyperplane and equidistant from it. These planes help in visualizing the margin, which is the distance between the support vectors and the hyperplane.
% Calculate margin planes
margin = 1 / norm(w);
y_neg = -(w(1) * x + b - margin) / w(2);
y_pos = -(w(1) * x + b + margin) / w(2);
% Plot margin planes
plot(x, y_neg, 'r--'); % Negative margin plane in red dashed line
plot(x, y_pos, 'g--'); % Positive margin plane in green dashed line
In this code, we calculate the margin using the norm of the weight vector w. The margin planes are then calculated and plotted using red and green dashed lines for the negative and positive margin planes, respectively.
Calculating the Maximum Margin
The maximum margin is twice the distance from the hyperplane to the margin planes. It represents the widest possible separation between the two classes.
% Calculate maximum margin
m = 2 * margin;
This simple line of code computes the maximum margin by multiplying the margin by two.
Displaying Results
Finally, we need to display the calculated hyperplane coefficients and the maximum margin in the required format. This is crucial for the assignment, as it allows you to present your results clearly and accurately.
% Display coefficients and margin
a = w(1);
b = w(2);
c = SVMModel.Bias;
fprintf('a=%.4f; b=%.4f; c=%.4f;\n', a, b, c);
fprintf('m=%.2f;\n', m);
Here, we extract the coefficients a, b, and c from the SVM model and print them in the specified format. The margin m is also printed in the required format.
Final MATLAB Script
Ensure your script is formatted correctly and includes all necessary parts. Here’s a sample structure of the complete script:
%% s1234567
AA = [9,9; 10,5; 16,8; 14,1; 15,2; 12,8; 12,2; 15,6; 10,12; 13,13];
BB = [-2,3; 1,6; 1,2; 2,4; -3,9; -1,5; -1,1; -5,6; -2,5; -4,3];
data = [AA; BB];
labels = [-1 * ones(size(AA, 1), 1); ones(size(BB, 1), 1)];
SVMModel = fitcsvm(data, labels, 'KernelFunction', 'linear', 'Standardize', true);
supportVectors = SVMModel.SupportVectors;
% Plotting
figure;
hold on;
scatter(AA(:,1), AA(:,2), 'r', 'filled');
scatter(BB(:,1), BB(:,2), 'g', 'filled');
scatter(supportVectors(:,1), supportVectors(:,2), 'ko', 'filled');
legend('Negative Class', 'Positive Class', 'Support Vectors');
title('2D Data Points for Two Classes');
xlabel('X-axis');
ylabel('Y-axis');
% Hyperplane and Margins
w = SVMModel.Beta;
b = SVMModel.Bias;
x = linspace(min(data(:,1)), max(data(:,1)), 100);
y = -(w(1) * x + b) / w(2);
margin = 1 / norm(w);
y_neg = -(w(1) * x + b - margin) / w(2);
y_pos = -(w(1) * x + b + margin) / w(2);
plot(x, y, 'b-');
plot(x, y_neg, 'r--');
plot(x, y_pos, 'g--');
hold off;
% Display results
a = w(1);
b = w(2);
c = SVMModel.Bias;
fprintf('a=%.4f; b=%.4f; c=%.4f;\n', a, b, c);
m = 2 * margin;
fprintf('m=%.2f;\n', m);
This final script includes all the steps we’ve discussed, from loading and plotting data to calculating and displaying the hyperplane and margin.
Conclusion
- Double-Check Data Entry: Ensure that your data is entered correctly to avoid any mistakes that could affect your results.
- Validate Results: Validate your results by visually inspecting the plot and ensuring the calculated hyperplane and margins align with the plotted data points.
- Review SVM Theory: Understanding the underlying theory of SVMs will help you make sense of the calculations and results.
By following these steps, you should be able to tackle similar SVM assignments effectively and efficiently using MATLAB. Happy coding!