Approaching Plane Elasticity with MATLAB from Discretization to Visualization
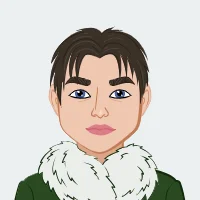
Understanding and solving plane elasticity problems is a crucial skill for engineering students, particularly those studying mechanical or civil engineering. These problems involve determining stress and strain in a material under load, which is fundamental in designing structures and materials that can withstand various forces and conditions. This blog will guide you through the process of solving similar MATLAB assignment, focusing on techniques that can be applied broadly rather than specific problem solutions.
Before diving into MATLAB coding, it's essential to understand the problem's context. Plane elasticity problems typically involve determining stress and strain in a material under load. For instance, consider a scenario where you have a material with specified boundary conditions, material properties (such as Young's modulus EEE and Poisson's ratio ν\nuν), and external forces.
Plane elasticity problems can be described by two-dimensional elasticity equations, which are derived from the general three-dimensional equations of elasticity. These equations take into account the balance of forces and compatibility of strains within the material.
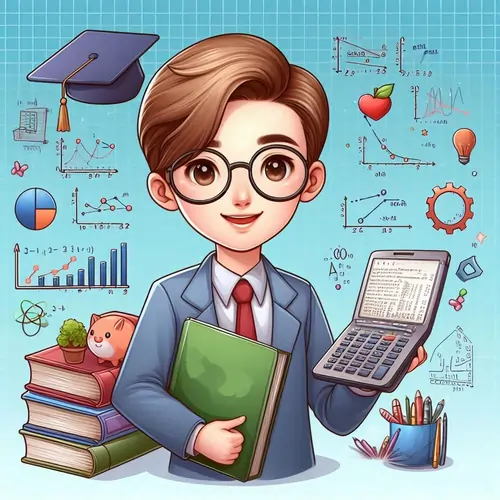
Key Concepts in Plane Elasticity
- Stress and Strain: Stress is the internal force per unit area within a material, while strain is the deformation or displacement per unit length.
- Young's Modulus (E): A measure of the stiffness of a material, defining the relationship between stress and strain.
- Poisson's Ratio (ν): A measure of the Poisson effect, where a material tends to expand in directions perpendicular to the direction of compression.
Step-by-Step Approach to Solving Plane Elasticity Problems
1. Problem Definition and Boundary Conditions
The first step in solving any plane elasticity problem is to define the geometry, boundary conditions, and material properties.
Geometry
Define the geometry of the problem. This includes specifying the dimensions and shape of the material under study. For instance, you might have a rectangular or triangular domain representing a section of a structural component.
Boundary Conditions
Identify the boundary conditions, which describe how the material is constrained or loaded. Boundary conditions can be:
- Displacement Boundary Conditions: Where specific displacements (e.g., fixed or zero displacement) are imposed on certain boundaries.
- Force Boundary Conditions: Where external forces or stresses are applied to the boundaries.
For example, in a plane elasticity problem, you might have a scenario where:
- Displacements uuu and vvv are fixed at x=0x = 0x=0.
- Certain edges are free of stresses.
- One edge has a distributed load applied.
2. Discretization of the Domain
Once the problem is defined, the next step is to discretize the domain into smaller elements. This process is known as meshing and is crucial for applying numerical methods such as the finite element method (FEM).
Types of Elements
- Linear Triangular Elements: Suitable for simpler problems and irregular geometries.
- Rectangular Elements: Can be used in combination with triangular elements for more complex problems.
In our example, we divide the domain into:
- Two linear triangular elements.
- A combination of a linear triangular element and a bi-linear rectangular element.
3. Formulating the Element Stiffness Matrix
The element stiffness matrix relates the nodal displacements to the nodal forces within each element. This matrix is derived using numerical methods, with the Galerkin method being a popular choice.
Galerkin Method
The Galerkin method involves approximating the solution by a set of basis functions and ensuring that the residuals (differences between the exact and approximate solutions) are orthogonal to the space spanned by these basis functions.
For each element, the local stiffness matrix (KeKeKe) and local force vector (FeFeFe) are derived.
4. Assembly of the Global Stiffness Matrix
After computing the local stiffness matrices and force vectors for each element, we assemble them into a global stiffness matrix (KKK) and a global force vector (FFF). This step involves adding the contributions from all elements to form the system of equations representing the entire domain.
Assembly Process
- Each element contributes to the global matrix and vector according to its position in the overall mesh.
- Ensure continuity and compatibility at the nodes shared by adjacent elements.
5. Applying Boundary Conditions
Boundary conditions are crucial for ensuring the solution's physical relevance. They modify the global stiffness matrix and force vector to reflect the constraints and loads applied to the system.
Applying Displacement Boundary Conditions
- Nodees with fixed displacements have their corresponding rows and columns in the stiffness matrix adjusted.
- The force vector is modified to account for prescribed displacements.
6. Solving the System of Equations
With the global stiffness matrix and force vector fully assembled and boundary conditions applied, we solve the linear system K⋅d=FK \cdot d = FK⋅d=F to find the displacement vector (ddd).
MATLAB Implementation
MATLAB’s built-in functions, such as the backslash operator (\), are efficient for solving systems of linear equations. The displacements at each node are obtained by solving this system.
7. Post-Processing the Results
The final step involves post-processing the results to extract meaningful information, such as stress and strain distributions, and visualize the solution.
Calculating Stress and Strain
- Use the displacement vector to compute strains and stresses at each node or element.
- Derive the stress components (σx\sigma_xσx, σy\sigma_yσy, and τxy\tau_{xy}τxy) from the strain components using Hooke’s law.
Visualization
MATLAB provides powerful tools for visualizing results. Functions like surf, quiver, and contour can be used to create plots of displacement fields, stress distributions, and other relevant quantities.
MATLAB Code Example
Here’s a basic outline of MATLAB code to solve a plane elasticity problem using the steps mentioned above:
% Define material properties
E = 210e9; % Young's modulus in Pa
nu = 0.3; % Poisson's ratio
% Define geometry and mesh
nodes = [...]; % Coordinates of nodes
elements = [...]; % Connectivity of elements
% Initialize global stiffness matrix and force vector
K = zeros(n_nodes); % Global stiffness matrix
F = zeros(n_nodes, 1); % Global force vector
% Loop over elements to assemble global stiffness matrix and force vector
for i = 1:n_elements
% Get element properties
element_nodes = elements(i, :);
coords = nodes(element_nodes, :);
% Calculate element stiffness matrix and force vector
[Ke, Fe] = element_stiffness(coords, E, nu);
% Assemble into global matrices
K = assemble_global(K, Ke, element_nodes);
F = assemble_global(F, Fe, element_nodes);
end
% Apply boundary conditions
[K_mod, F_mod] = apply_boundary_conditions(K, F, boundary_conditions);
% Solve for displacements
displacements = K_mod \ F_mod;
% Post-processing: Calculate stresses and strains
stresses = calculate_stresses(nodes, elements, displacements, E, nu);
% Visualization
plot_results(nodes, elements, displacements, stresses);
Detailed Explanation of the Code
- Define Material Properties: The Young’s modulus (EEE) and Poisson’s ratio (ν\nuν) are essential for calculating the stiffness matrix.
- Define Geometry and Mesh: The coordinates of the nodes and the connectivity of the elements define the domain's discretization.
- Initialize Global Matrices: The global stiffness matrix (KKK) and force vector (FFF) are initialized to zeros.
- Assemble Global Matrices: Loop through each element, calculate the local stiffness matrix and force vector, and assemble them into the global matrices.
- Apply Boundary Conditions: Modify the global matrices to incorporate boundary conditions.
- Solve for Displacements: Use MATLAB’s linear solver to find the displacement vector.
- Post-Processing: Calculate stresses and strains based on the displacements and visualize the results.
Tips for Similar Assignments
Understand the Theory
A solid understanding of the underlying theory of elasticity and numerical methods like the Galerkin method is crucial. This theoretical foundation ensures that you can derive the necessary equations and matrices correctly.
Utilize MATLAB Functions
MATLAB offers a range of functions that simplify various tasks, from creating meshes to solving linear systems and visualizing results. Functions like linspace, meshgrid, surf, quiver, and contour are particularly useful.
Write Modular Code
Writing modular code with functions for each task (e.g., stiffness matrix calculation, assembly, boundary condition application) makes your program more manageable and reusable. It also simplifies debugging and allows for easy modifications and extensions.
Verification and Validation
Always verify your results with known solutions or simpler problems to ensure correctness. Validation against analytical solutions or benchmark problems helps build confidence in your numerical solution.
Conclusion
Solving plane elasticity problems using MATLAB involves several steps: understanding the problem, discretizing the domain, formulating and assembling matrices, applying boundary conditions, solving the equations, and post-processing the results. By following these steps and utilizing MATLAB's powerful computational capabilities, you can tackle similar assignments efficiently. This approach not only helps in solving specific problems but also equips you with a methodology applicable to a wide range of engineering challenges.
Understanding the nuances of plane elasticity and mastering the use of MATLAB for such problems will significantly enhance your problem-solving skills in engineering. This guide provides a structured approach that you can adapt and expand upon, ensuring you are well-prepared for a variety of related assignments and real-world applications.