Applied Parallel Computing on Modern Supercomputers: A MATLAB Approach
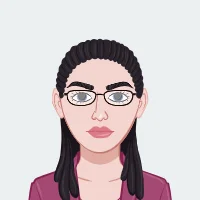
Parallel computing is integral to modern computational science and engineering, essential for leveraging the increasing power of supercomputers to efficiently solve complex problems. This blog provides a comprehensive introduction to applied parallel computing using MATLAB, aiming to equip students with foundational concepts and practical approaches. By understanding the principles, learners can enhance their ability to solve MATLAB assignment in fields ranging from scientific simulations to data analysis. MATLAB’s Parallel Computing Toolbox enables parallel execution of tasks, optimizing performance through concurrent processing of computational tasks. Through this guide, students will grasp key concepts such as concurrency, parallelism, and scalability, crucial for dividing large tasks into smaller, manageable units that execute simultaneously. As they explore numerical and geometrical topics like dense and sparse linear algebra, N-body simulations, and mesh generation, they will gain insights into optimizing algorithms for supercomputing architectures. Ultimately, mastering these techniques empowers students to harness the full potential of parallel computing, advancing their proficiency in MATLAB and tackling complex computational challenges effectively.
Understanding Parallel Computing
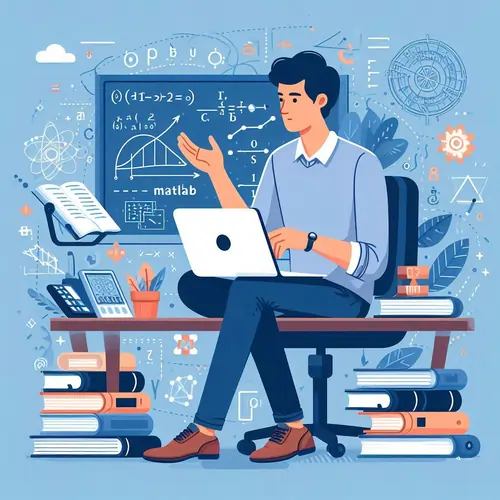
Parallel computing involves dividing a large computational task into smaller, independent tasks that can be executed simultaneously. This approach significantly reduces computation time and allows for solving problems that would otherwise be infeasible.
Key Concepts
- Concurrency vs. Parallelism: Concurrency involves multiple tasks making progress simultaneously, whereas parallelism specifically refers to tasks running at the same time.
- Granularity: The size of tasks into which a problem is decomposed. Fine-grained tasks are small and numerous, while coarse-grained tasks are larger and fewer.
- Scalability: The ability of an algorithm or program to efficiently utilize an increasing number of processors.
Numerical Topics in Parallel Computing
Dense and Sparse Linear Algebra
Linear algebra is a cornerstone of many scientific computations. Understanding how to perform these operations in parallel can lead to significant performance improvements.
Dense Linear Algebra: Involves operations on matrices that are fully populated with non-zero elements. Use libraries like MATLAB’s Parallel Computing Toolbox for matrix operations.
- Matrix Multiplication: Perform large matrix multiplications in parallel using parfor and spmd commands.
- Eigenvalue Problems: Solve eigenvalue problems using parallel algorithms to reduce computation time.
Sparse Linear Algebra: Deals with matrices that contain a significant number of zero elements. Exploit sparsity to reduce computational complexity and memory usage. MATLAB functions like spmd and parfor can be useful.
- Sparse Matrix Operations: Utilize sparse matrices to save memory and increase computation speed for large-scale problems.
- Conjugate Gradient Method: Implement the conjugate gradient method in parallel to solve large sparse systems of linear equations.
N-body Problems
N-body problems involve calculating the interactions between a large number of particles, such as gravitational forces in astrophysics or molecular dynamics simulations.
Divide and Conquer: Break down the problem into smaller subsets that can be solved independently and then combined.
- Barnes-Hut Algorithm: Use the Barnes-Hut algorithm to approximate N-body interactions, reducing the complexity from O(N^2) to O(N log N).
- Parallel Tree Construction: Implement parallel algorithms to construct spatial trees, enhancing performance for large datasets.
Fast Multipole Methods (FMM): Reduce the complexity of N-body simulations by approximating interactions between distant particles.
- Multipole Expansion: Apply multipole expansions to group distant particles and reduce the number of direct interactions.
- Parallel FMM Implementation: Use parallel programming techniques to implement FMM, leveraging MATLAB’s parallel capabilities.
Multigrid Methods
Multigrid methods accelerate the solution of large linear systems arising from discretized partial differential equations.
V-Cycle and W-Cycle: Understand the different cycling strategies in multigrid methods to optimize performance.
- Multigrid V-Cycle: Implement the V-Cycle approach to solve linear systems efficiently.
- Multigrid W-Cycle: Utilize the W-Cycle method for problems requiring higher accuracy.
Coarse and Fine Grids: Solve the problem on a hierarchy of grids, from coarse to fine, to enhance convergence rates.
- Grid Transfer Operations: Implement efficient grid transfer operations between coarse and fine grids.
- Parallel Multigrid Solvers: Develop parallel multigrid solvers to exploit the full potential of modern supercomputers.
Geometrical Topics in Parallel Computing
Partitioning and Mesh Generation
Effective partitioning and mesh generation are critical for the performance of parallel finite element and finite volume methods.
Domain Decomposition: Divide the computational domain into subdomains that can be processed in parallel.
- Static Partitioning: Use static partitioning techniques to divide the domain before computation begins.
- Dynamic Partitioning: Implement dynamic partitioning to adjust subdomains during computation for load balancing.
Load Balancing: Ensure that each processor has an approximately equal amount of work to avoid idle times.
- Load Balancing Algorithms: Apply load balancing algorithms to distribute computational workload evenly.
- Parallel Mesh Refinement: Develop parallel mesh refinement techniques to enhance accuracy and efficiency.
Parallel Programming Paradigms
Message Passing Interface (MPI)
MPI is a standard for distributed memory parallel computing. It allows processes to communicate with one another by sending and receiving messages.
Point-to-Point Communication: Direct communication between two processes.
- MPI Send/Receive: Use MPI_Send and MPI_Recv functions for basic point-to-point communication.
- Non-blocking Communication: Implement non-blocking communication to overlap computation and communication, enhancing performance.
Collective Communication: Communication involving a group of processes, such as broadcasting or gathering data.
- MPI_Bcast: Broadcast data from one process to all other processes using MPI_Bcast.
- MPI_Reduce: Use MPI_Reduce to perform reduction operations, such as summing values from all processes.
Data Parallel Systems
Data parallelism involves distributing data across different parallel computing nodes, with each node performing the same operation on its portion of the data.
Vectorization: Use MATLAB’s built-in vectorized operations to exploit data parallelism.
- Array Operations: Perform element-wise operations on arrays using vectorized code.
- Matrix Functions: Utilize MATLAB’s matrix functions to operate on entire matrices in parallel.
MapReduce: Implement the MapReduce programming model for processing large datasets.
- Map Function: Define a map function to process data in parallel.
- Reduce Function: Use a reduce function to aggregate results from the map phase.
Star-P for Parallel Python and Parallel MATLAB
Star-P provides an interactive parallel computing environment, enabling the use of parallel MATLAB and Python.
Interactive Development: Develop and debug parallel applications interactively.
- Parallel MATLAB Functions: Use Star-P to execute MATLAB functions in parallel across multiple processors.
- Python Integration: Integrate Python code with Star-P to leverage parallel computing capabilities.
Seamless Integration: Combine MATLAB and Python code within a parallel computing framework.
- Hybrid Programming: Develop hybrid programs that utilize both MATLAB and Python for different parts of the computation.
- Performance Optimization: Optimize performance by choosing the most suitable language and parallel strategy for each task.
Advanced Topics
Graphics Processors (GPUs)
GPUs offer massive parallelism for certain types of computations, particularly those involving large-scale matrix operations and image processing.
CUDA and OpenCL: Understand the basics of GPU programming using CUDA and OpenCL.
- CUDA Kernels: Write CUDA kernels to perform parallel computations on the GPU.
- OpenCL Programs: Develop OpenCL programs to run on a variety of parallel computing devices.
MATLAB GPU Computing: Utilize MATLAB functions like gpuArray and arrayfun to offload computations to the GPU.
- GPU Arrays: Create and manipulate GPU arrays for parallel computation.
- Custom GPU Functions: Write custom GPU functions using arrayfun to enhance performance.
Virtualization
Virtualization allows multiple virtual machines to run on a single physical machine, each with its own operating system and resources.
Containerization: Use Docker and other container technologies to create reproducible and portable computing environments.
- Docker Containers: Develop and deploy parallel applications in Docker containers for consistency and portability.
- Kubernetes: Use Kubernetes for orchestration and management of containerized parallel applications.
Hypervisors: Learn about different types of hypervisors and their impact on performance.
- Type 1 Hypervisors: Use Type 1 hypervisors for direct access to hardware resources and improved performance.
- Type 2 Hypervisors: Utilize Type 2 hypervisors for development and testing on virtual machines.
Caches and Vector Processors
Efficient use of memory hierarchy and vector processing units can greatly enhance the performance of parallel applications.
Cache Optimization: Implement strategies to minimize cache misses and maximize data locality.
- Blocking Techniques: Use blocking techniques to enhance cache performance for matrix operations.
- Prefetching: Implement prefetching to load data into the cache before it is needed.
SIMD Instructions: Use Single Instruction, Multiple Data (SIMD) instructions to perform parallel operations on vectors of data.
- Vectorization: Leverage SIMD instructions for vectorized operations in MATLAB.
- Performance Tuning: Tune performance by optimizing memory access patterns and reducing branching.
Conclusion
Applied parallel computing on modern supercomputers offers immense potential for solving complex scientific and engineering problems. By understanding and implementing the techniques discussed in this blog, such as parallel linear algebra, N-body simulations, multigrid methods, and effective partitioning, you can harness the power of parallel computing in your MATLAB assignments. Additionally, exploring advanced topics like GPU computing, virtualization, and cache optimization will further enhance your ability to tackle challenging computational tasks efficiently.