Morse Code Translation and Visualization in MATLAB
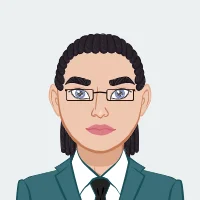
MATLAB is a versatile tool for various engineering and scientific applications, and one fascinating task it can handle is translating Morse code to English and visualizing the result in a pie chart. This blog provides a comprehensive guide to solving similar assignments, offering detailed instructions and best practices to help students master these concepts effectively. The blog contents will be beneficial for students also who want to complete their Data Analysis assignment in MATLAB .
Setting Up Your MATLAB Environment
The first step in tackling any MATLAB assignment is setting up your environment properly. This ensures your code runs smoothly and without unexpected issues. Here are the essential commands to start with:
clear all; close all;
The clear all command clears all variables from the workspace, ensuring no residual data interferes with your current session. The close all command closes all figure windows, giving you a clean slate for your graphical outputs.
Defining the Morse Code Conversion Chart
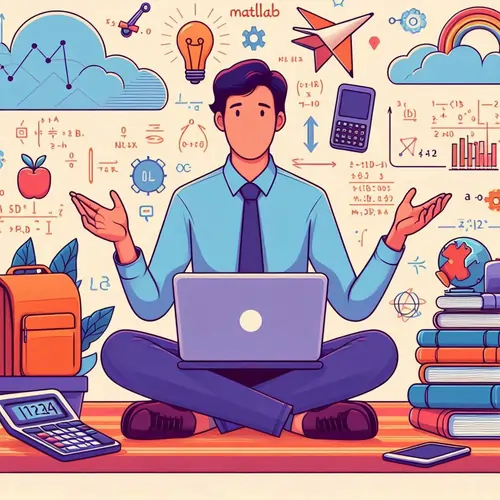
A critical component of this assignment is the Morse code conversion chart. This chart maps Morse code symbols to their corresponding English letters and numbers. In MATLAB, we can represent this chart using two arrays: one for Morse code symbols and one for the corresponding letters.
Here's how you can define these arrays:
code = [".----", "..---", "...--", "....-", ".....", "-....", "--...", "---..", "----.", "-----", ...
".-", "-...", "-.-.", "-..", ".", "..-.", "--.", "....", "..", ".---", "-.-", ".-..", "--", ...
"-.", "---", ".--.", "--.-", ".-.", "...", "-", "..-", "...-", ".--", "-..-", "-.--", "--..", ...
"..--.-"];
letter = ["1", "2", "3", "4", "5", "6", "7", "8", "9", "0", "A", "B", "C", "D", "E", "F", "G", "H", ...
"I", "J", "K", "L", "M", "N", "O", "P", "Q", "R", "S", "T", "U", "V", "W", "X", "Y", "Z", ...
"-"];
Translating Morse Code to Text
The next step is to create a function that translates Morse code into text. This function will take a string of Morse code and convert it into its corresponding English representation.
Here's a function that performs this translation:
function translatedText = translateMorse(morseCode, code, letter)
morseSymbols = strsplit(morseCode, '/');
translatedText = '';
for i = 1:length(morseSymbols)
index = find(strcmp(morseSymbols{i}, code));
if ~isempty(index)
translatedText = [translatedText, letter{index}];
else
translatedText = [translatedText, '?'];
end
end
end
In this function, strsplit is used to split the Morse code string into individual symbols using the slash ("/") as a delimiter. The strcmp function compares each Morse symbol to the code array, and the corresponding letter is appended to the translated text.
Counting Letter Frequencies
Once the Morse code has been translated into text, the next step is to analyze the frequency of each letter. This involves counting how often each letter appears in the translated text.
Here’s a function to count the frequency of each letter:
function letterFreq = countLetterFrequency(translatedText, letter)
letterFreq = zeros(1, length(letter));
for i = 1:length(translatedText)
index = find(translatedText(i) == letter);
if ~isempty(index)
letterFreq(index) = letterFreq(index) + 1;
end
end
end
This function initializes a frequency array with zeros and then iterates through the translated text, incrementing the count for each letter.
Visualizing Data with a Pie Chart
Visualization is an important aspect of data analysis. In this assignment, you need to create a pie chart that shows the frequency of each letter in the translated text.
Here’s how you can create a pie chart in MATLAB:
function plotPieChart(letterFreq, letter)
figure;
pie(letterFreq, letter);
title('Letter Frequency in Translated Morse Code');
end
The pie function creates a pie chart, and the title function adds a title to the chart. The letterFreq array contains the frequencies, and the letter array contains the corresponding labels.
Integrating Everything in the Main Script
To complete the assignment, you need to integrate all the functions into a main script that executes the translation and visualization.
Here’s an example of the main script:
% Main Script
clear all; close all;
code = [".----", "..---", "...--", "....-", ".....", "-....", "--...", "---..", "----.", "-----", ...
".-", "-...", "-.-.", "-..", ".", "..-.", "--.", "....", "..", ".---", "-.-", ".-..", "--", ...
"-.", "---", ".--.", "--.-", ".-.", "...", "-", "..-", "...-", ".--", "-..-", "-.--", "--..", ...
"..--.-"];
letter = ["1", "2", "3", "4", "5", "6", "7", "8", "9", "0", "A", "B", "C", "D", "E", "F", "G", "H", ...
"I", "J", "K", "L", "M", "N", "O", "P", "Q", "R", "S", "T", "U", "V", "W", "X", "Y", "Z", ...
"-"];
morseCode = "-.-./---/...-/../-../.----/----./..--.- /.--./.-/-./-.././--/../-.-./..--.- /...-/.-/-.-./-.-./../-././";
translatedText = translateMorse(morseCode, code, letter);
letterFreq = countLetterFrequency(translatedText, letter);
plotPieChart(letterFreq, letter);
This script starts by clearing the workspace and closing all figure windows. It then defines the Morse code and letter arrays, translates the Morse code, counts the letter frequencies, and plots the pie chart.
Best Practices for MATLAB Coding
To ensure your code is effective and easy to maintain, follow these best practices:
1. Code Commenting
Good commenting is crucial for understanding and maintaining your code. Aim to comment at least 20% of your lines with useful information that explains the logic and functionality of your code.
2. Use of Functions
Modularize your code by breaking it down into functions. This not only makes your code more readable but also allows for easier debugging and testing. In this assignment, we created separate functions for translating Morse code, counting letter frequencies, and plotting the pie chart.
3. Code Formatting
Maintain proper indentation and formatting throughout your code. This improves readability and helps you identify the structure and flow of your code. Avoid nested functions if possible and minimize code redundancy by using arrays and loops effectively.
4. Testing
Thoroughly test your code with various Morse code inputs to ensure it handles different scenarios correctly. This includes testing with valid Morse code strings, empty strings, and invalid symbols. Testing helps you identify and fix any potential issues before submission.
Applying These Concepts to Other Assignments
The skills and techniques discussed in this blog can be applied to a wide range of MATLAB assignments. Whether you’re working on signal processing, data analysis, or visualization tasks, the following steps will help you approach any assignment methodically:
Step 1: Understand the Requirements
Carefully read the assignment instructions to understand the objectives and requirements. Identify the key tasks you need to perform and the expected outputs.
Step 2: Break Down the Problem
Divide the assignment into smaller, manageable tasks. This makes it easier to focus on one aspect at a time and ensures you don’t overlook any important steps.
Step 3: Plan Your Solution
Before writing any code, plan your approach. Outline the main functions you need to implement and the logic for each. This helps you stay organized and ensures you cover all the necessary steps.
Step 4: Implement and Test
Implement your solution step by step, testing each part as you go. This iterative approach helps you catch errors early and ensures your code works as expected.
Step 5: Document Your Code
Comment your code thoroughly and include documentation that explains how to run your script and what each function does. This is especially important for assignments that will be graded or reviewed by others.
Step 6: Review and Optimize
Once your code is complete, review it for any potential improvements. Look for ways to optimize performance, reduce redundancy, and improve readability.
Conclusion
Solving MATLAB assignments involving Morse code translation and visualization requires a combination of problem-solving skills, coding proficiency, and attention to detail. By following the steps outlined in this blog, you can tackle similar assignments with confidence and develop a strong foundation in MATLAB programming.
Remember to maintain clean and well-documented code, test your solutions thoroughly, and apply these best practices to other assignments. With practice and perseverance, you’ll be able to handle complex MATLAB tasks and achieve success in your coursework.