How to Use MATLAB to Solve Assignments on Fuzzy Logic Controllers
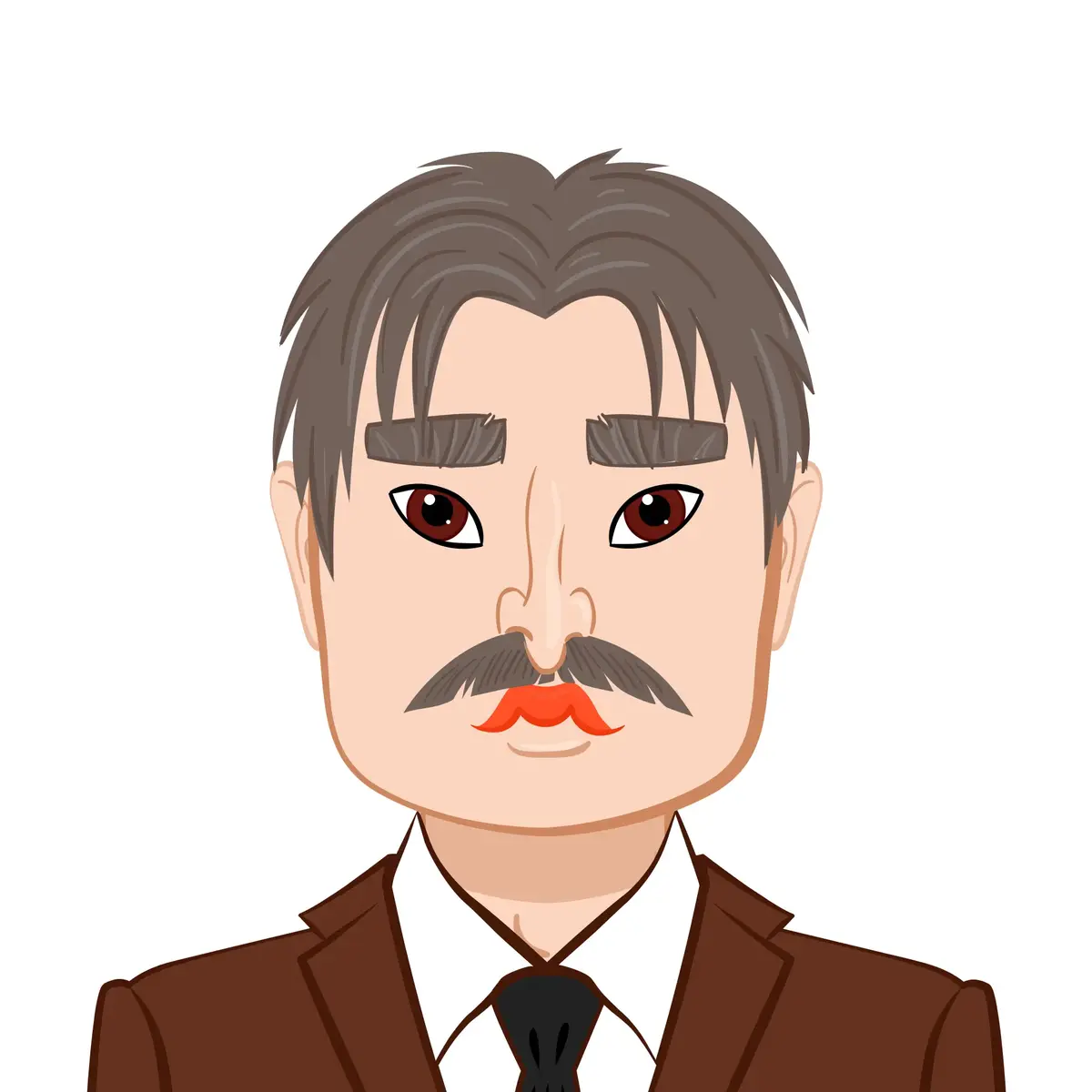
Fuzzy Logic Controllers (FLC) are widely used in industries and research due to their ability to handle uncertainty and non-linearity in systems. As a student working with FLCs, you will likely need to understand both the theoretical aspects and practical application of these controllers. MATLAB, a powerful computational tool, provides a comprehensive environment for developing, testing, and simulating fuzzy logic-based systems. If you find yourself struggling with the complexity of FLCs, seeking assistance with fuzzy logic assignment can be a valuable way to gain deeper insights and better understand the material. In this blog, we'll guide you through the process of using MATLAB to solve assignments on Fuzzy Logic Controllers, combining both theory and hands-on examples to help you master this topic.
What is a Fuzzy Logic Controller (FLC)?
Fuzzy logic is an extension of classical logic that allows reasoning with imprecise, uncertain, or approximate information. In traditional Boolean logic, variables can only take the values of true or false (1 or 0), but fuzzy logic allows variables to take any value between 0 and 1, providing a way to deal with the vagueness and ambiguity in real-world scenarios. A Fuzzy Logic Controller is a control system that uses fuzzy logic to control the behavior of a system.
FLCs consist of several components:
- Fuzzification: This process converts crisp input values into fuzzy values using membership functions.
- Rule Base: A set of fuzzy rules that define the system's behavior.
- Inference Engine: The core of the FLC that applies the fuzzy rules to generate output fuzzy values.
- Defuzzification: The process of converting fuzzy output values back into crisp values to make the final decision.
In MATLAB, the Fuzzy Logic Toolbox provides tools for designing and simulating FLCs.
MATLAB's Fuzzy Logic Toolbox
MATLAB provides an intuitive interface and a wide range of functions to model, analyze, and simulate fuzzy logic controllers. The Fuzzy Logic Toolbox allows you to design FLCs through graphical interfaces and MATLAB code. The toolbox supports creating fuzzy systems, defining membership functions, setting up rule bases, and running simulations.
Creating a Fuzzy Inference System (FIS)
A FIS is the structure that represents a fuzzy logic system. In MATLAB, you can create a FIS using the fis command or the graphical interface.
1. Using the MATLAB Command Line
To create a fuzzy system, you can define a mamfis (Mamdani-type fuzzy inference system) object. Here is an example of creating a simple FIS with two input variables and one output variable:
% Create the fuzzy inference systemfis = mamfis('NumInputs', 2, 'NumOutputs', 1);% Define the input variablesfis = addInput(fis, [0 10], 'Name', 'Temperature');fis = addInput(fis, [0 10], 'Name', 'Pressure');% Define the output variablefis = addOutput(fis, [0 10], 'Name', 'FanSpeed');% Define membership functions for the inputs and outputfis = addMF(fis, 'Temperature', 'trapmf', [0 0 3 6], 'Name', 'Low');fis = addMF(fis, 'Temperature', 'trapmf', [3 6 10 10], 'Name', 'High');fis = addMF(fis, 'Pressure', 'trapmf', [0 0 4 7], 'Name', 'Low');fis = addMF(fis, 'Pressure', 'trapmf', [4 7 10 10], 'Name', 'High');fis = addMF(fis, 'FanSpeed', 'trimf', [0 5 10], 'Name', 'Medium');
This code creates a fuzzy system with two inputs, Temperature and Pressure, and one output, FanSpeed. The inputs are defined as trapezoidal membership functions, while the output is defined as a triangular membership function.
2. Using the Graphical Interface
MATLAB also provides a graphical interface called the Fuzzy Logic Designer that allows you to visually design fuzzy inference systems. To open it, type the following command in MATLAB:
fuzzyLogicDesigner
This interface allows you to:
- Create fuzzy systems by adding inputs, outputs, and defining membership functions.
- Set up rules and visualize the control surface.
- Simulate and test the system.
Setting Up Rules and Simulating FLCs
Once the fuzzy system is created, the next step is to define the rules that determine how the system will respond to various input combinations. Rules are generally written in the form of IF-THEN statements, such as:
- IF Temperature is High AND Pressure is Low THEN FanSpeed is High.
- IF Temperature is Low AND Pressure is High THEN FanSpeed is Low.
In MATLAB, you can add rules to the fuzzy system using the addrule function:
% Add fuzzy rulesrule1 = [1 1 1 1 1]; % IF Temp is Low AND Pressure is Low THEN FanSpeed is Mediumrule2 = [2 2 1 1 1]; % IF Temp is High AND Pressure is High THEN FanSpeed is Highfis = addrule(fis, [rule1; rule2]);
After defining the rules, you can simulate the fuzzy system with specific input values using the evalfis function:
% Simulate the FLC for specific inputsinputs = [5 7]; % Example input values: Temperature = 5, Pressure = 7output = evalfis(fis, inputs);disp(['The fan speed is: ', num2str(output)]);
This will calculate the FanSpeed based on the inputs and rules you defined.
Advanced Techniques for Fuzzy Logic Control
Once you are familiar with basic fuzzy logic control, you can explore more advanced topics such as fuzzy PID controllers, optimization techniques, and fuzzy logic with neural networks.
Fuzzy PID Control
A fuzzy PID controller combines traditional PID control with fuzzy logic to improve performance in systems where a standard PID controller might struggle due to uncertainties. MATLAB allows you to design fuzzy PID controllers using the Fuzzy Logic Toolbox and Simulink.
Implementing Fuzzy PID Control in MATLAB
You can implement a fuzzy PID controller by combining a fuzzy logic system for the control input and a standard PID controller for the output. Here's a basic structure:
- Define Fuzzy Logic Rules: Use fuzzy inputs like error and change in error to adjust the PID gains.
- Tuning the PID Controller: Use fuzzy logic to modify the proportional, integral, and derivative gains dynamically based on the system's state.
Using Fuzzy Logic with Neural Networks
Neural networks can be used alongside fuzzy logic to enhance the performance of control systems, particularly in systems where the relationships between variables are highly nonlinear. MATLAB supports integrating fuzzy logic with neural networks to create hybrid models.
Example: Combining Fuzzy Logic and Neural Networks
% Create and train a neural networknet = feedforwardnet(10); % Example: 10 neurons in the hidden layernet = train(net, inputs, targets);% Use fuzzy logic to adjust the neural network outputs dynamicallyoutput = evalfis(fis, input);adjusted_output = net(output); % Adjust output based on neural network
This technique can be used for advanced control systems requiring adaptive and nonlinear behavior.
Tips for Solving Fuzzy Logic Controller Assignments Using MATLAB
To effectively use MATLAB to solve assignments involving Fuzzy Logic Controllers, here are some practical tips:
1. Understand the Assignment Requirements
Before you start coding in MATLAB, thoroughly understand the problem statement. Identify the inputs, outputs, and the type of FLC required (Mamdani or Sugeno). This will help you structure your FIS correctly.
2. Break Down the Problem into Steps
Work in smaller increments. Start by defining the fuzzy system and membership functions, then proceed to define the rules, simulate the system, and validate the output. This modular approach will make debugging and troubleshooting easier.
3. Use MATLAB’s Built-in Functions
MATLAB has several built-in functions like mamfis, addInput, addOutput, addMF, and addrule, which simplify the process of defining and testing fuzzy systems. Don't reinvent the wheel—leverage these functions to save time and reduce errors.
4. Test with Different Inputs
Once your FLC is designed, test it with various input combinations to ensure it behaves as expected. This will help you identify any errors in your rules or membership functions.
5. Visualize the System
Use MATLAB's plotting functions to visualize the membership functions and the surface plots of your fuzzy system. This will help you understand the behavior of your FLC and make adjustments as needed.
% Visualize the membership functionsplotmf(fis, 'input', 1);plotmf(fis, 'output', 1);
6. Use Simulink for Complex Systems
For complex FLCs or systems requiring dynamic simulations, consider using Simulink. The Fuzzy Logic Toolbox integrates with Simulink, allowing you to build dynamic models that can be simulated in real-time.
Conclusion
MATLAB is a powerful tool for solving assignments related to Fuzzy Logic Controllers, providing both theoretical and practical support. By creating fuzzy inference systems, defining membership functions and rules, and simulating the system, students can tackle FLC assignments effectively. If you ever find yourself struggling with complex aspects of fuzzy logic, seeking help with Matlab assignment can make a big difference. Advanced topics such as fuzzy PID control and integrating fuzzy logic with neural networks further enhance the capabilities of MATLAB in solving real-world control problems. With practice, you will become proficient in using MATLAB to design, simulate, and analyze fuzzy logic-based systems for your assignments and research projects.