Efficient Techniques to Solve MATLAB Assignments on Fourier Transforms and Simulink
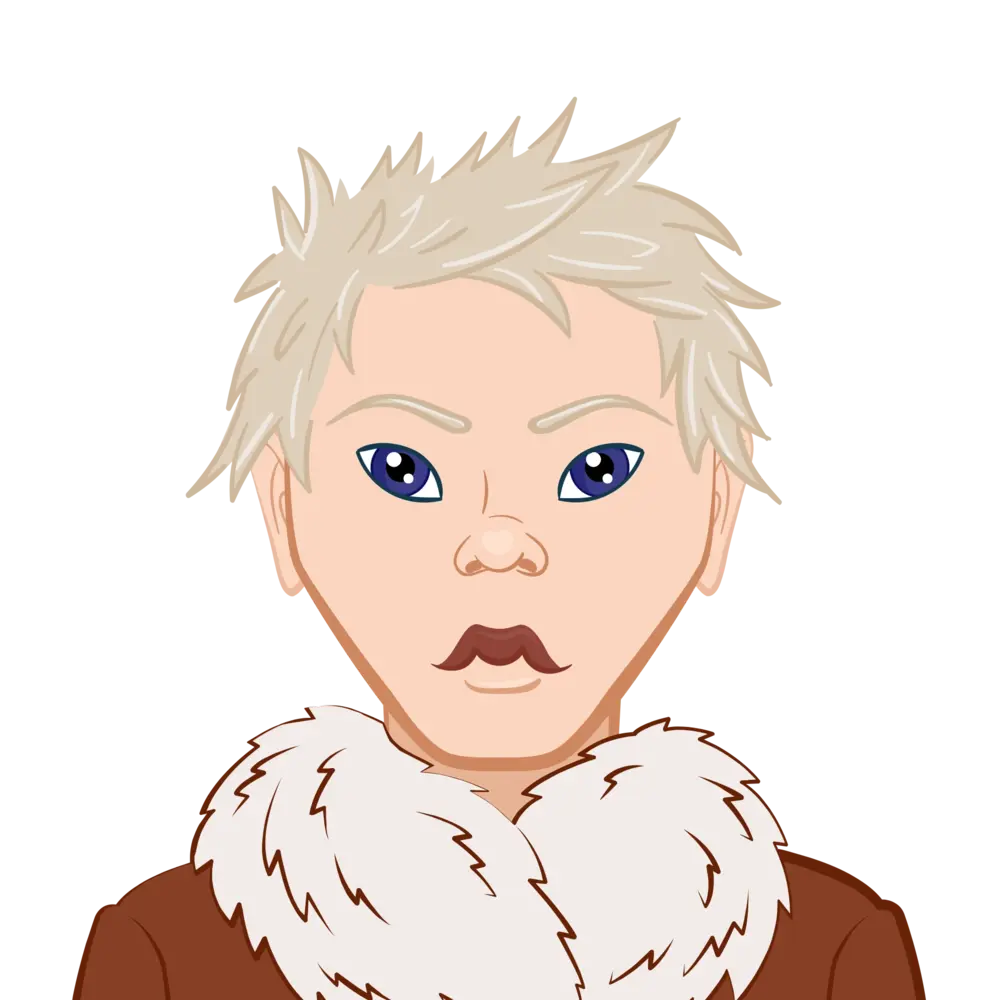
MATLAB is an essential tool in engineering and scientific research, particularly in fields like signal processing, control systems, and mechanical systems modeling. Among its many features, MATLAB excels in the analysis and simulation of systems using Fourier Transforms and Simulink. Fourier Transforms are key for analyzing signals in the frequency domain, making them indispensable for tasks such as signal filtering, compression, and noise removal. Simulink, a MATLAB-based graphical programming environment, is widely used for modeling, simulating, and analyzing dynamic systems.
When tackling assignments that involve these topics, students and professionals can benefit from a structured approach. The key six steps will efficiently help to solve your MATLAB assignment on Fourier Transforms and Simulink are: understanding the problem, setting up the MATLAB environment, defining the problem mathematically, implementing the solution using MATLAB code or Simulink blocks, analyzing the results, and refining the model. By following these steps, users can not only achieve accurate results but also gain a deeper understanding of the underlying concepts, ultimately making complex assignments more manageable. This blog provides valuable insights into these steps, offering practical advice to enhance both learning and practical application of MATLAB in the context of Fourier Transforms and Simulink simulations.
Step 1: Symbolic Fourier Transforms
In this first step, you will need to use MATLAB’s symbolic math toolbox to find the Fourier transform of different input signals. The Fourier Transform is a critical concept in signal processing that allows us to analyze the frequencies that make up a signal.
a) Finding the Fourier Transform
To begin, you will define your input signal as a symbolic function in MATLAB. You will then apply the Fourier Transform to the signal. The symbolic Fourier Transform can be computed using MATLAB's fourier function. For instance:
syms t f
x = cos(2*pi*t); % Define a simple cosine signal
X = fourier(x); % Compute the Fourier Transform
disp(X)
This code will return the Fourier Transform of the input signal x, which is a representation in the frequency domain.
b) Simplification
After obtaining the Fourier Transform, it's crucial to simplify the result wherever possible. MATLAB’s simplify function helps in reducing the complexity of symbolic expressions. For example:
X_simplified = simplify(X);
disp(X_simplified)
This will give a simpler form of the Fourier Transform, which is more manageable and easier to interpret.
c) Inverse Fourier Transform
The inverse Fourier Transform is used to recover the time-domain signal from its frequency-domain representation. In MATLAB, the ifourier function is used to perform this operation. Here’s an example:
x_inverse = ifourier(X_simplified);
disp(x_inverse)
This step completes the transformation from the frequency domain back to the time domain, and you can now visualize how the signal looks in the time domain.
Step 2: Investigating Audio Recordings of a Piano with a Discrete Fourier Transform
Next, you will apply the Discrete Fourier Transform (DFT) to audio signals, particularly to analyze the frequency components of piano notes. The DFT helps in breaking down the sound into its constituent frequencies, allowing you to identify the fundamental frequency and the overtones (harmonics).
1. Loading Audio Files
Before analyzing the signal, you must load the audio files into MATLAB. Here’s how you can load the piano sound recordings:
load('pianoSounds.mat'); % Loads the audio data
This command loads the audio files, which are stored as vectors in the workspace. The note1, note2, and note3 variables contain the recordings of individual notes, while chord contains a chord made up of multiple notes played simultaneously.
2. Listening to the Audio Files
To listen to the recordings, use MATLAB’s audioplayer function:
p = audioplayer(note1, fs); % fs is the sampling frequency
play(p); % Play the note
You can replace note1 with other notes (e.g., note2 or note3) to listen to the different sounds.
3. Time Domain Plots
To understand the signal better, it's essential to plot the audio data in the time domain. MATLAB allows you to create multiple subplots for easy visualization. You can plot the signals for different time windows to observe the variations in the audio signal over time:
subplot(1,3,1);
plot(t, note3);
xlim([0 2.2]); % Set the time axis limits
title('Time Domain: Full Signal');
subplot(1,3,2);
plot(t, note3);
xlim([1 1.2]); % Zoomed-in view
title('Time Domain: Zoomed-in Signal');
subplot(1,3,3);
plot(t, note3);
xlim([1 1.005]); % Further zoomed-in view
title('Time Domain: Further Zoomed-in Signal');
By analyzing the plots, you’ll notice periodic patterns that correspond to the frequencies of the notes.
4. Discrete Fourier Transform of the Notes
Now, the goal is to analyze the frequencies present in each note using the Discrete Fourier Transform (DFT). Use MATLAB's fft() function to compute the DFT of the audio signals:
Y1 = fft(note1);
Y2 = fft(note2);
Y3 = fft(note3);
f = (0:length(Y1)-1)*(fs/length(Y1)); % Frequency vector
subplot(1,2,1);
plot(t, note1);
title('Time Domain: Note 1');
subplot(1,2,2);
plot(f, abs(Y1)); % Plot the magnitude of the Fourier coefficients
title('Frequency Domain: Note 1');
xlim([0 2500]); % Frequency range for analysis
The magnitude of the DFT shows the strength of different frequencies in the signal. By analyzing these peaks, you can identify the fundamental frequency of the note and the presence of overtones.
Step 3: Analyzing a Chord with DFT
When multiple notes are played together, such as in a chord, their frequencies blend, and analyzing the chord in the time domain becomes challenging. The solution is to apply the DFT to the chord and identify the individual notes.
1. Time Domain Analysis of the Chord
To understand how the chord looks in the time domain, plot the signal with an appropriate time window:
subplot(1,2,1);
plot(t, chord);
xlim([0.5 0.53]);
title('Time Domain: Chord');
The time-domain plot of a chord appears much more complex than individual notes. However, applying the DFT can help separate the individual components.
2. Frequency Domain Analysis of the Chord
Perform the DFT of the chord and plot the amplitude spectrum:
Y_chord = fft(chord);
subplot(1,2,2);
plot(f, abs(Y_chord));
title('Frequency Domain: Chord');
xlim([0 2500]);
By analyzing the peaks in the frequency domain, you can identify the frequencies corresponding to the individual notes in the chord.
Step 4: Synthesizing an Artificial Note with Sinusoids and Envelope Modulation
To understand the synthesis of musical notes, you will now synthesize an artificial piano note using sinusoids. A real piano note has a complex waveform consisting of a fundamental frequency and multiple harmonics. By combining sinusoids, we can approximate this note.
1. Synthesize a Single Sinusoidal Note
Start by approximating the piano note with a single sinusoidal signal at the fundamental frequency:
t = 0:1/fs:2.2; % Define the time vector
f0 = 440; % Frequency of the note (e.g., A4 = 440 Hz)
note_synth = sin(2*pi*f0*t); % Create a pure sinusoidal note
This synthetic note will have the same frequency as the piano note but will lack the richness provided by the overtones.
2. Add Envelope Modulation
Real piano notes have a characteristic attack and decay pattern. You can simulate this by applying an envelope to the signal. Use the given envelope data:
note_synth_enveloped = note_synth .* envelope; % Apply the envelope modulation
This step adds volume modulation to the signal, making it sound more natural.
3. Add Harmonics to the Synthesis
To make the synthetic note sound more realistic, add overtones based on the frequency analysis from Step 2. For each harmonic, use the frequency and amplitude data from the DFT to create additional sinusoids:
harmonics = sum(sin(2*pi*f_harmonics*t)); % Sum over the harmonic frequencies
note_synth_realistic = note_synth_enveloped + harmonics;
This note will now have a more realistic timbre, similar to a real piano note.
Step 5: System Response Analysis Using Symbolic Fourier Transforms
The fifth step focuses on analyzing systems using symbolic Fourier transforms. In engineering and control systems, this technique is useful for understanding the frequency response of systems. You can define the system's input and transfer functions symbolically and calculate the output in the frequency domain.
syms w
H = transfer_function(w); % Define the system's transfer function
F = input_signal(w); % Define the input signal
X = F * H; % Multiply input by transfer function in frequency domain
This symbolic approach allows you to examine how the system responds to various frequencies.
Step 6: Application of Simulink for System Simulation
Finally, Simulink is a powerful tool in MATLAB for simulating dynamic systems. You can use it to simulate and analyze systems such as mechanical, electrical, or control systems.
1. Create a Simulink Model
In Simulink, you can create a model that represents the system you want to analyze. Drag and drop blocks to represent components such as transfer functions, integrators, and sum blocks to model the dynamics of your system.
2. Simulate the System
Once the model is created, run the simulation to observe the system's behavior. You can analyze the output in both the time and frequency domains using the same techniques as in earlier steps.
sim('model_name'); % Run the Simulink model
The results from Simulink will help you understand how your system behaves under various conditions.
Conclusion
By following these six steps, you'll unlock MATLAB’s full potential for signal processing and system analysis. The journey starts with understanding symbolic Fourier transforms and how they can simplify complex mathematical problems. MATLAB’s symbolic toolbox allows you to compute transforms symbolically, enhancing your grasp of the underlying concepts. Next, the blog dives into practical applications, such as using Simulink for system modeling and simulation. By setting up dynamic simulations, you can visualize and test the behavior of systems in real-time, refining your approach to solve your Simulink assignment related problems. Additionally, MATLAB’s extensive plotting and data visualization features help you analyze and present your results clearly. With these techniques, you’ll develop the confidence to tackle advanced assignments, whether in academics or professional work. Overall, MATLAB’s capabilities offer a comprehensive suite of tools to efficiently solve your Fourier Transforms assignment and Simulink, ensuring your success in the field.