Approach to Solve MATLAB Assignments on Chemical Engineering and Advanced Control Systems
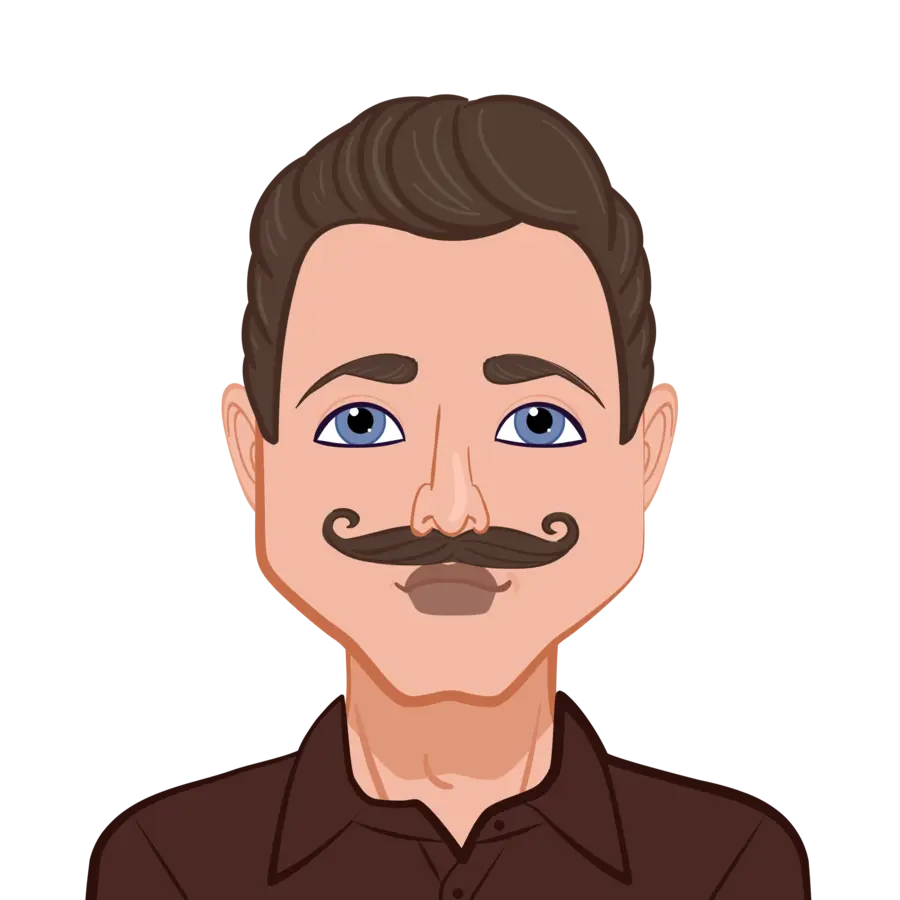
MATLAB is a powerful computational tool widely used in engineering, especially in chemical engineering and control systems. Many students struggle with advanced control assignments involving modeling, thermodynamic calculations, and system identification due to the complexity of iterative methods and property calculations. Understanding how to systematically approach such assignments is crucial for solving them efficiently. This blog provides a structured guide to solve your MATLAB assignment on advanced control systems and thermodynamics. Key steps include defining the problem, identifying required equations, selecting appropriate numerical methods, and implementing the solution in MATLAB. Techniques such as Newton’s method, fsolve, and Antoine’s equation for vapor pressure calculations are commonly used in solving thermodynamic equilibrium problems. Additionally, working with MATLAB functions and scripts helps in modularizing complex calculations.
Students should also validate results by cross-checking with theoretical values and debugging errors systematically. Efficient coding practices, such as vectorization and the use of built-in MATLAB functions, enhance accuracy and speed. By following these strategies, students can develop confidence to solve their control system assignment and chemical engineering using Matlab. With practice, MATLAB can become a valuable tool for solving real-world engineering problems efficiently.
Step 1: Understand the Problem and Break It Down
Before diving into coding, the first and most important step is understanding the problem at hand. Often, assignments can seem overwhelming because they are filled with complex equations, data, and conditions. However, by breaking the problem down into smaller, manageable parts, you can make the task more approachable. For example, in an assignment that involves a liquid mixture, you may need to calculate thermodynamic properties like bubble point temperature, vapor pressure, and molar density. These individual calculations can be tackled separately and combined in the final program.
One important consideration is to understand the underlying principles of the problem. For example, let’s say you're given a mixture of chemicals (e.g., ethane, propylene, propane, etc.) and asked to calculate the bubble point temperature at a certain pressure. The core concept here is to balance the vapor pressure of the mixture with the mole fractions of each component. Breaking it down into smaller tasks like this can help ensure you don't get lost in the complexity of the assignment.
To help you get started, break the problem into clear sub-tasks such as:
- Defining the input parameters like mole fractions, pressures, and Antoine constants.
- Setting up equations that describe the system's behavior, such as those for vapor pressure and bubble point temperature.
- Writing functions that will help calculate thermodynamic properties.
- Using numerical solvers to solve for unknowns in the system.
Step 2: Mathematical Formulation and Assumptions
Once you've broken the problem down, the next step is to translate it into mathematical equations. Often, chemical engineering assignments require using well-known equations to describe the physical processes, such as Antoine’s equation for vapor pressures or formulas to calculate the molar density and enthalpy of liquid and vapor mixtures.
In the case of calculating bubble point temperature, the equation you'll typically encounter is:
Where:
- Psat(T) is the saturation pressure of each component at temperature T,
- xj is the mole fraction of each component in the mixture,
- P is the total pressure of the system.
This equation essentially balances the total vapor pressure of the mixture with the mole fractions, which will lead to the bubble point temperature when solved.
It is essential to understand the assumptions you are making while formulating the model. For example:
- Assume ideal behavior of the liquid mixture, meaning Raoult's law applies (which states that the vapor pressure of each component is proportional to its mole fraction in the liquid phase).
- Assume that the system is at equilibrium.
These assumptions will help simplify the problem and allow you to apply standard thermodynamic equations to solve for the unknowns.
Step 3: MATLAB Program Structure
Now that you have formulated the problem and have a clear understanding of the equations, it's time to translate your approach into MATLAB code. MATLAB is a versatile programming language used for numerical computing, which makes it an ideal tool for solving engineering problems. It allows you to perform matrix operations, work with functions, and solve complex equations efficiently.
In MATLAB, you often break the problem into smaller sub-programs, which can be functions or scripts. Each function should focus on solving one specific part of the problem. For example, if you need to calculate the vapor pressure of each component in the mixture, you can write a function that takes temperature as input and returns the vapor pressure using Antoine’s equation.
Here is a general structure for the MATLAB code you would write to solve the bubble point temperature calculation:
% Define given data such as mole fractions, pressure, etc.
xj = [0.03, 0.4, 0.15, 0.15, 0.27]; % Mole fractions of components
P = 2.4e6; % Total pressure in Pascals (2.4 MPa)
% Antoine constants for each compound (example values)
A = [8.07131, 8.07260, 8.06307, 8.02999, 8.09599]; % Antoine constants A
B = [1730.63, 1724.69, 1751.60, 1750.14, 1752.78]; % Antoine constants B
C = [233.426, 233.211, 235.050, 233.331, 234.046]; % Antoine constants C
% Define the Antoine equation for vapor pressure calculation
antione_eqn = @(T, A, B, C) 10.^(A - (B / (T + C)));
% Define the function for calculating bubble point temperature
bubble_point = @(T) sum(xj .* antione_eqn(T, A, B, C) / P) - 1;
% Initial guess for bubble point temperature (in Kelvin)
T_guess = 300; % Starting guess for temperature
% Solve using fsolve
options = optimset('Display', 'iter'); % Display iteration details
T_bubble = fsolve(bubble_point, T_guess, options); % Solve for bubble point temperature
disp(['Bubble Point Temperature: ', num2str(T_bubble), ' K']);
Step 4: Validate Your Results
After writing the code, the next step is to test it with known values to ensure that the results are accurate. Validation is crucial because it helps you confirm that the program is working as expected. For example, you could compare your calculated bubble point temperature with values from published sources or hand-calculated estimates to see if the results are reasonable.
When testing, try using simple test cases that you can easily calculate by hand, or compare your results with benchmarks from textbooks or research papers. If your program gives results that are close to these benchmarks, you can be confident that the program works as intended.
Step 5: Handle Special Considerations and Exceptions
While solving these problems, you may encounter some special conditions that you need to account for. For example:
- Convergence Issues: Numerical solvers like fsolve might not converge, especially if the initial guess is far from the true solution. In this case, try adjusting the initial guess or using a different numerical method.
- Realistic Values: Always check that the values of physical properties such as pressure, temperature, and densities are within reasonable physical limits. This can help avoid any unrealistic results.
- Dimensional Consistency: Ensure that your equations are dimensionally consistent. For example, if you are working with mole fractions, make sure all units in the equations are compatible with the system of units you are using.
Step 6: Optimization and Documentation
Once your program is working, focus on optimizing and documenting the code. Optimization could involve improving the speed of numerical calculations, especially if the problem involves large datasets or complex systems. You can also improve the readability of your code by structuring it well and adding comments to explain what each part of the code does.
Here are some tips for documentation:
- Use comments liberally to explain what each function does and what each variable represents.
- If your program contains functions, clearly explain the inputs, outputs, and any assumptions that each function makes.
- Ensure that your final program is clean, well-organized, and easy to follow.
Conclusion
MATLAB is an indispensable tool for solving complex problems in chemical engineering and control systems. By following a structured approach—understanding the problem, formulating it mathematically, implementing it in MATLAB, validating your results, and considering special conditions—you can confidently tackle any similar assignment. This approach not only helps you solve the task at hand but also provides you with the skills to handle future assignments efficiently. Whether you're calculating bubble point temperatures, molar densities, or enthalpies, MATLAB can simplify and accelerate the process, allowing you to focus on understanding the principles behind the equations.
If you ever find yourself struggling with an assignment or need extra guidance on MATLAB programming for control systems or chemical engineering, don’t hesitate to seek help. By mastering the approach described above, you will not only complete your assignments more efficiently but also gain valuable problem-solving skills that will serve you throughout your academic and professional career.