How to Tackle and Solve MATLAB Assignments Using Finite Element Method (FEM)
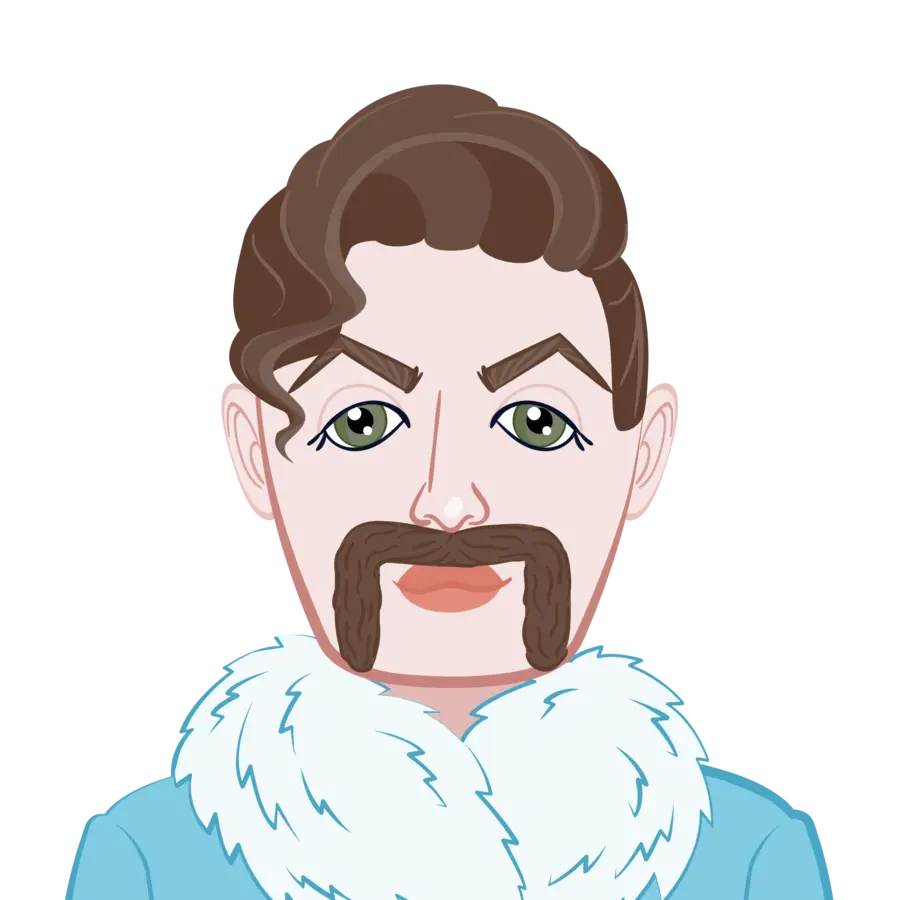
When faced with an assignment involving the Finite Element Method (FEM) and MATLAB, students often encounter challenges in understanding both the theory and the coding required to solve such problems. The assignment you’ve mentioned revolves around the modelling of axial vibrations of rods using FEM, eigenvalue problems, and dynamic simulations in MATLAB. Although the specifics of each assignment may differ, the underlying principles and approaches are often similar. This guide will walk you through how to solve your Matlab assignment using FEM that involve axial vibrations, system dynamics, and more.
1. Understanding the Problem Statement
The first step in any FEM assignment is to understand the problem thoroughly. In this case, the task involves two rods with different properties colliding and vibrating axially together. The problem asks for the calculation of the first natural frequency of the combined system using FEM. Although this problem involves complex dynamics, the underlying steps to solve it are based on understanding how FEM works, how to apply it in MATLAB, and how to interpret the results.
Key concepts to focus on:
- Finite Element Method (FEM): FEM is a numerical technique used for solving differential equations by dividing the problem domain into smaller subdomains (elements). These elements are then analyzed individually, and the results are combined to approximate the solution to the overall problem.
- Eigenvalue Problems: In the context of vibrations, eigenvalues correspond to the natural frequencies of the system, and eigenvectors describe the mode shapes.
- Axial Vibrations: These are vibrations that occur along the length of a rod or beam. In this assignment, the motion is constrained to the axial direction, which simplifies the analysis.
2. Breaking Down the Assignment into Smaller Steps
For any complex FEM assignment, breaking it down into smaller manageable tasks is crucial. In this case, the problem can be divided into the following components:
- Preprocessing:
- Defining the material properties (density, Young's modulus, etc.)
- Defining the geometric properties (length, cross-section, etc.)
- Discretizing the rod into finite elements
- Modeling the System:
- Setting up the stiffness matrix and mass matrix for each rod
- Assembling the global system matrix for the combined rods
- Solving the Eigenvalue Problem:
- Applying boundary conditions and solving for the first natural frequency
- Time Response Analysis (Dynamic Simulation):
- Solving the system of differential equations using MATLAB’s ode45 solver
- Plotting the displacement and velocity at various points in time
- Postprocessing:
- Analyzing and interpreting the results (natural frequencies, displacement-time history, etc.)
Each of these steps requires both theoretical understanding and practical application of MATLAB coding skills.
3. Preprocessing: Setting Up the Problem in MATLAB
The first step is defining all the parameters necessary for your system. This includes the material properties, the geometry of the rods, and the number of elements used for discretization.
- Material Properties:
- For rod I, you will have properties such as the length (L1), side of the square cross-section (a1), density (rho1), and Young’s modulus (E1). Similarly, rod II has its own set of material properties.
- These properties need to be entered into MATLAB, either manually or by creating arrays or matrices.
- Geometry:
- The geometry involves defining the rod lengths, cross-sectional areas, and dividing the rods into finite elements. The number of elements for each rod is specified in the problem (e.g., N1 = 16 for rod I and N2 = 24 for rod II).
- Discretization:
- Discretizing the rods into finite elements involves dividing the length of each rod into smaller segments. The nodes at each end of these segments will be used to define the degrees of freedom.
In MATLAB, you can define these properties using variables:
L1 = 0.8; % length of rod I
L2 = 1.2; % length of rod II
a1 = 0.08; % cross-sectional area of rod I
a2 = 0.07; % cross-sectional area of rod II
rho1 = 4540; % density of rod I
rho2 = 2700; % density of rod II
E1 = 110e9; % Young's modulus of rod I (Pa)
E2 = 69e9; % Young's modulus of rod II (Pa)
4. Modeling the System: Constructing Stiffness and Mass Matrices
The next step is to model the system using FEM. This involves constructing the stiffness matrix (K) and mass matrix (M) for each rod. For each finite element, the stiffness and mass matrices can be calculated based on the material properties, geometry, and the number of elements.
- Stiffness Matrix (K): This matrix represents the resistance of the system to deformation.
- Mass Matrix (M): This matrix represents the inertia of the system, determining how the system responds to applied forces.
For a truss element, the local stiffness matrix for an element in the axial direction can be expressed as:
k_local = (E * A / L) * [1, -1; -1, 1];
Where:
- E is the Young’s modulus,
- A is the cross-sectional area,
- L is the length of the element.
Once the local stiffness matrices are calculated, they can be assembled into a global stiffness matrix that represents the entire system. Similarly, the mass matrix can be calculated using similar principles.
5. Solving the Eigenvalue Problem
Once the stiffness and mass matrices are constructed, the next step is solving for the natural frequencies of the combined system. This involves solving an eigenvalue problem of the form:
[K - lambda * M] * phi = 0
Where:
- K is the global stiffness matrix,
- M is the global mass matrix,
- lambda is the eigenvalue (corresponding to the square of the natural frequency),
- phi is the eigenvector (mode shape).
In MATLAB, you can solve for the natural frequencies using the eig function:
[mode_shapes, natural_frequencies] = eig(K, M);
The output will give you the mode shapes and natural frequencies of the system. The first natural frequency is often the most important, especially when analyzing the vibrations of a structure.
6. Time Response Analysis: Using MATLAB’s ode45 Solver
Once the natural frequencies are found, the next step is to simulate the time response of the system. This involves solving the second-order ordinary differential equations (ODEs) that govern the motion of the rods after collision.
For this, MATLAB provides the ode45 solver, which is ideal for solving non-stiff ODEs. The general form of the ODE for the system can be written as:
M * u''(t) + K * u(t) = F(t)
Where:
- M is the mass matrix,
- K is the stiffness matrix,
- u(t) is the displacement vector,
- F(t) is the applied force vector.
To use ode45, you will need to define the initial conditions for the system, such as initial displacements and velocities, and then define the ODE function. Here’s an example of how you can set it up:
initial_conditions = [u0; v0]; % initial displacement and velocity
[t, u] = ode45(@(t, u) odefun(t, u, M, K), t_span, initial_conditions);
Where odefun is a function that defines the system of ODEs, and t_span is the time interval over which to solve the system.
7. Postprocessing: Analyzing the Results
After solving the ODEs, the next step is to analyze the results. MATLAB allows you to plot the displacement-time history of the system, which is essential for understanding the dynamic behavior of the rods.
To plot the displacement of the free tip, you can use:
plot(t, u(:,end)); % Displacement of the last node over time
xlabel('Time (s)');
ylabel('Displacement (m)');
This will give you a time history plot showing how the displacement of the free tip of the rod changes with time.
8. Interpreting the Results and Making Calculations
Once you have obtained the displacement-time data, you will need to interpret it to answer specific questions in the assignment, such as calculating the displacement at a specific time or comparing it with the expected values. You can use simple MATLAB commands like min, max, or interp1 to extract the relevant data points.
For example, to calculate the displacement at a particular time, say tQ = 0.001s, you can use:
u_at_tQ = interp1(t, u(:,end), 0.001);
Final Thoughts
By following these steps, you will be well-prepared to tackle similar assignments involving vibration analysis and FEM in MATLAB. The key is to break down the problem into manageable steps—starting with modeling the system, followed by calculating the stiffness and mass matrices, solving for natural frequencies, simulating the dynamic response, and finally validating your results through post-processing and comparison to expected values.
The process might seem complex at first, but with practice and familiarity with MATLAB, it becomes much easier to approach these types of problems. Always ensure that you understand the underlying physics and mathematics before diving into the coding part, and make use of MATLAB's extensive documentation and resources to help you along the way.