How to Write Efficient MATLAB Assignment Code Using Loops and Conditional Logic
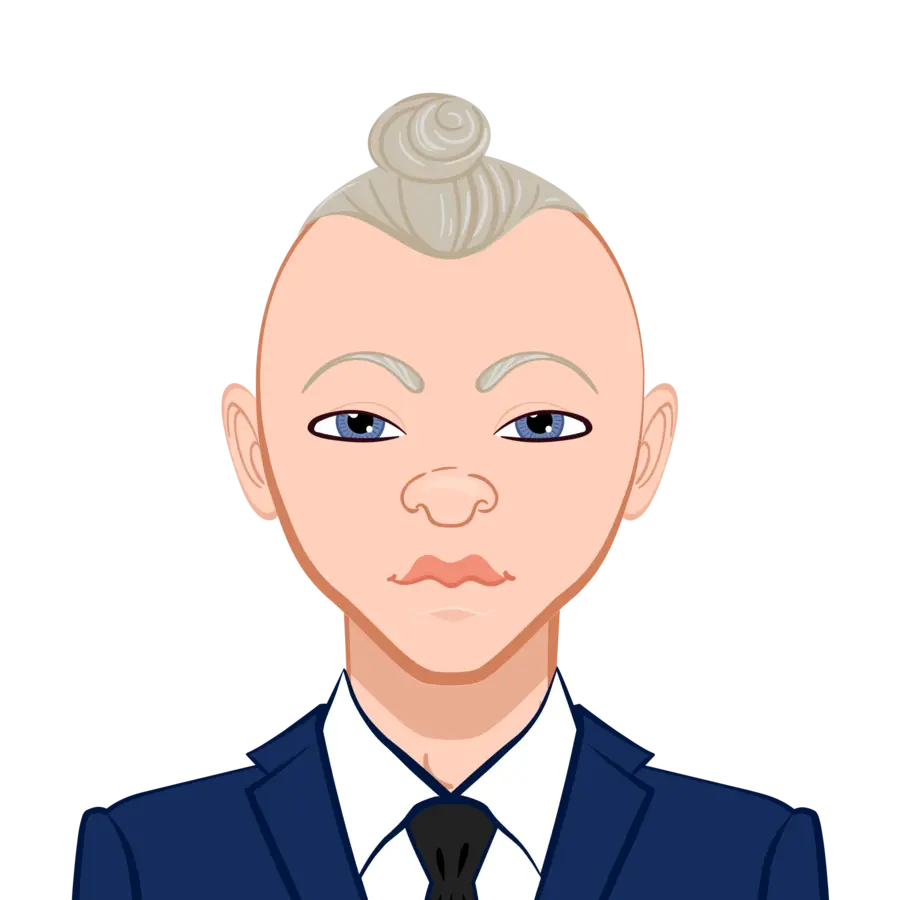
MATLAB, short for Matrix Laboratory, is a high-level programming language and interactive environment used by engineers, scientists, and researchers for numerical computation, data analysis, and algorithm development. Students working on MATLAB assignments, you may encounter problems that involve basic elements of programming, such as loops, conditional statements, and functions. Understanding how to efficiently use these elements is key to solving a variety of MATLAB problems, regardless of the specific task at hand. This guide aims to help you master these concepts so you can approach to complete your MATLAB assignment with confidence.
Understanding the Problem and Breaking It Down
The first step in solving any MATLAB assignment is to carefully read and understand the problem statement. Whether you’re tasked with writing code for numerical analysis, data manipulation, or creating a graphical user interface (GUI), the concepts of loops, conditional statements, and functions are likely to play a key role.
Let’s begin by understanding how these elements fit into the broader context of MATLAB assignments.
- Input Data: Often, you will need to ask the user for data. MATLAB provides tools to handle different types of user inputs, such as numeric values, strings, and arrays.
- Loops: Many MATLAB assignments involve loops, especially when working with vectors and matrices. Loops allow you to iterate through elements in an array or perform repetitive tasks until a certain condition is met.
- Conditional Statements: These are used when you need to make decisions in your code. For example, if certain criteria are met, you might execute a set of commands; otherwise, you might execute a different set of commands.
- Functions: Functions are reusable pieces of code that perform specific tasks. By writing functions, you can keep your code modular and clean, which is especially helpful for more complex assignments.
By understanding how these elements work individually and together, you can apply them to a wide range of problems. Let’s dive deeper into how you can approach these elements in MATLAB.
1. Getting Comfortable with User Input in MATLAB
A common task in MATLAB assignments is to ask users for input. Understanding how to use the input() function will help you gather data from the user and incorporate it into your program.
Using the input() Function
MATLAB provides the input() function, which allows you to prompt the user for data and store that data in a variable. The syntax is straightforward:
x = input('Enter a number: ');
This code will display a message asking the user to enter a number. Once the user enters a value, MATLAB will store it in the variable x.
For more complex data types, such as vectors or matrices, the input() function can also handle these. For instance, if your assignment requires the user to enter a vector, you can use the following code:
vec = input('Enter a vector: ');
The user would need to input a vector in the form of [1, 2, 3, 4] or similar. For a matrix, the input would be something like [1, 2; 3, 4], representing a 2x2 matrix.
It is important to provide instructions to the user about what type of input is expected. For instance, if the program expects a numeric value, it may be useful to add a message saying “Enter a numeric value” to avoid confusion.
Validating User Input
In some cases, it’s important to validate the input to ensure it meets specific criteria. For example, if your assignment expects only positive numbers, you could use a while loop to prompt the user for input until they provide a valid response:
x = input('Enter a positive number: ');
while x <= 0
disp('The number must be positive. Please try again.');
x = input('Enter a positive number: ');
end
In this case, if the user enters a number less than or equal to zero, the program will prompt them again until they enter a valid positive number.
2. Using Loops for Vectors and Matrices
One of the most powerful features in MATLAB is its ability to efficiently manipulate vectors and matrices. Loops are a fundamental programming structure that allows you to automate repetitive tasks, such as iterating through elements in a vector or performing calculations on each element of a matrix.
For Loops in MATLAB
A for loop is used when you know in advance how many times you need to iterate. The basic syntax for a for loop is:
for i = startValue:endValue
% Code to execute during each iteration
End
For example, if you need to iterate through a vector and display each element, you can use a for loop like this:
vec = [1, 2, 3, 4, 5]; % Example vector
for i = 1:length(vec)
disp(vec(i)); % Display each element of the vector
end
In this case, the loop will iterate from 1 to the length of the vector, and during each iteration, it will display the value of the current element.
While Loops in MATLAB
A while loop is useful when you don’t know in advance how many times you need to repeat an action, but rather when a certain condition is met. The basic syntax is:
while condition
% Code to execute while the condition is true
End
For example, you might want to sum the elements of a vector until a certain condition is met, such as a sum exceeding a given threshold. You could use a while loop for this:
vec = [1, 2, 3, 4, 5];
sumVec = 0;
i = 1;
while sumVec < 10
sumVec = sumVec + vec(i);
i = i + 1;
end
disp(['Sum exceeds 10: ', num2str(sumVec)]);
In this case, the loop will continue iterating until the sum of the elements exceeds 10.
3. Implementing Conditional Statements
Conditional statements allow your program to make decisions based on specific conditions. These are crucial when you need to execute different actions depending on the values of variables.
Using if, else, and elseif Statements
MATLAB supports several types of conditional statements, the most common being if, else, and elseif. Here’s a simple example using these statements:
x = input('Enter a number: ');
if x > 0
disp('Positive number');
elseif x < 0
disp('Negative number');
else
disp('Zero');
end
This code will display whether the number entered by the user is positive, negative, or zero. The elseif clause is useful when you need to check multiple conditions.
Nested Conditional Statements
Sometimes, you need to check multiple conditions within each other. You can use nested if statements to handle such scenarios. For example:
x = input('Enter a number: ');
if x > 0
if mod(x, 2) == 0
disp('Positive even number');
else
disp('Positive odd number');
end
elseif x < 0
disp('Negative number');
else
disp('Zero');
end
In this example, after checking if x is positive, the program further checks if the number is even or odd using the mod() function.
4. Functions to Simplify Your Code
Functions allow you to organize your code into manageable blocks, making it reusable and easier to maintain. Functions are particularly useful when solving assignments that require repetitive tasks or need specific actions to be performed in multiple places in your program.
Creating and Using Functions
To define a function in MATLAB, use the following syntax:
function output = functionName(input)
% Code to perform task
output = result;
end
For example, let’s say you need to check whether a number is positive, negative, or zero multiple times in your program. Instead of repeating the same code, you can create a function:
function checkNumber(x)
if x > 0
disp('Positive number');
elseif x < 0
disp('Negative number');
else
disp('Zero');
end
end
Now you can call this function whenever you need to check a number:
checkNumber(5); % Output: Positive number
checkNumber(-3); % Output: Negative number
checkNumber(0); % Output: Zero
Returning Multiple Outputs
If you need to return multiple values from a function, MATLAB allows you to do so using square brackets. For example:
function [sumResult, productResult] = calculateSumAndProduct(x, y)
sumResult = x + y;
productResult = x * y;
end
You can then call this function and capture both outputs:
[sumVal, productVal] = calculateSumAndProduct(2, 3);
disp(['Sum: ', num2str(sumVal), ', Product: ', num2str(productVal)]);
5. Debugging and Testing Your Code
Once you’ve written your code, it’s essential to test it with various inputs to ensure that it behaves as expected. MATLAB’s debugging tools allow you to step through your code line-by-line, examine variable values, and identify errors.
Use the Editor in MATLAB to set breakpoints, which will pause execution at specific points in your code. From there, you can step through each line and inspect the current state of your variables. This will help you identify where things might be going wrong.
Conclusion
In conclusion, loops, conditional statements, and functions are the backbone of MATLAB programming. By understanding how to work with these fundamental elements, you’ll be equipped to solve a wide range of assignments. Whether you’re dealing with vectors, matrices, or complex expressions, these tools will enable you to write clean, efficient, and maintainable code. Practice regularly, break down problems into smaller steps, and always test your code to ensure its accuracy. With time and effort, you’ll become proficient in solving MATLAB assignments and applying your knowledge to real-world problems.