How to Tackle MATLAB Assignments Using Data Analysis and Visualization
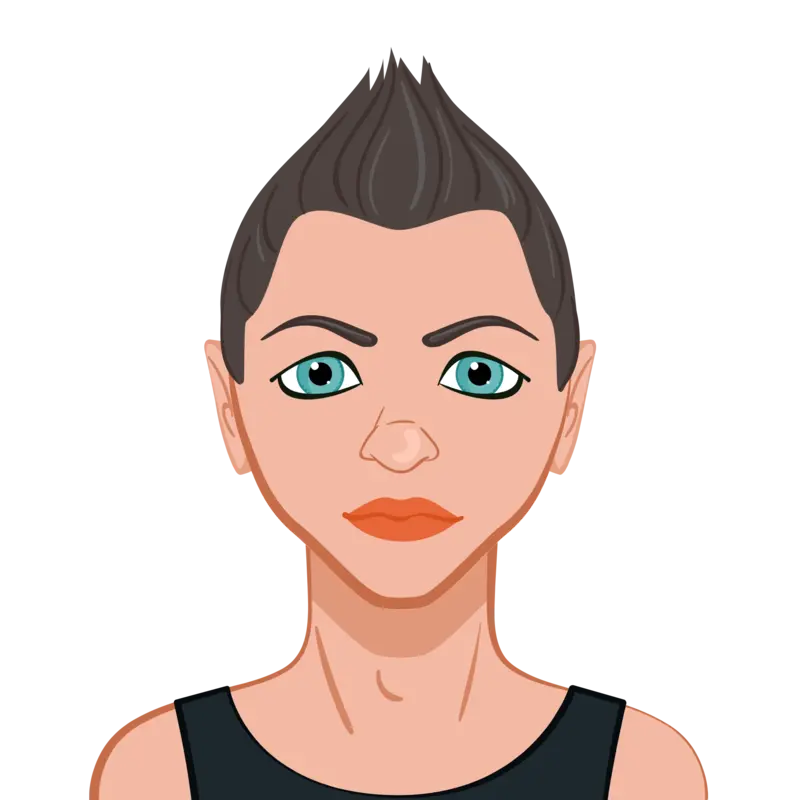
MATLAB is a vital tool for students in engineering, data science, and mathematics, offering robust capabilities for data analysis, computation, and visualization. Whether you're new to MATLAB or have prior experience, understanding how to approach assignments effectively is key to success. This guide explores a comprehensive method for tackling MATLAB assignments, using an example involving data from an electric vehicle (EV) trip. The blog covers tasks such as importing data, processing and converting measurements, and visualizing results. It demonstrates how to analyze electric vehicle performance, power consumption, and battery temperature while emphasizing the importance of clean, well-commented code. These techniques not only help to solve their data analysis assignment but also build a strong foundation for handling data visualization challenges in the future.
By mastering the steps of data import, analysis, and visualization, students can confidently approach MATLAB assignments across diverse fields. This guide equips with practical strategies to enhance problem-solving skills, apply computational methods, and create effective visualizations, ensuring you achieve clarity and accuracy in your work. While focused on EV data, the approaches here are adaptable, making them a valuable resource for any MATLAB assignment.
Step 1: Understand the Assignment Requirements
Before diving into the code, it's essential to read and understand the assignment requirements. A typical MATLAB assignment includes several tasks where students must manipulate data, perform mathematical computations, and present their findings visually. For example, an assignment might require you to analyze the performance of an electric vehicle, such as the Nissan Leaf, by processing data from the car's sensors. The task may involve extracting data, converting units, performing calculations (e.g., power consumption), and plotting various variables (e.g., speed, battery voltage, temperature).
To break this down:
- Data Loading – You’ll typically need to import data from a file format like CSV or Excel.
- Data Conversion – Some assignments require unit conversions, such as converting miles per hour to kilometers per hour or volts to kilovolts.
- Mathematical Computations – You may need to compute quantities like battery power, motor power, or temperature statistics.
- Visualization – Most assignments require plotting your results, such as plotting power consumption against time or displaying the distribution of battery temperatures across sensors.
As you read through the assignment, underline the key instructions, and identify the tasks that require coding. Understanding the scope of the assignment is crucial for efficiently planning your approach.
Step 2: Load and Import Data into MATLAB
The first task in most MATLAB assignments is to load data into the environment. MATLAB offers several functions to import data from different file formats. For example, if your assignment involves a CSV file containing sensor data from a Nissan Leaf, you would likely use the readtable() or csvread() function to import the data.
Here's a typical example of how to load data from a CSV file:
% Import data from CSV file
data = readtable('Log_U0006387_160311740d0.csv');
Once the data is loaded, it’s a good practice to check the first few rows to verify the import:
% Inspect the first few rows of the data
head(data);
This will display the first few rows of your dataset, allowing you to confirm that the data was loaded correctly and that the columns match the expected format. At this stage, it's also essential to check the supplementary documentation, such as the Log File Format.docx, to understand the meaning of each data column and any necessary units.
For example, the data might include the following variables:
- Time (in seconds)
- Speed (in miles per hour or kilometers per hour)
- Gear
- Elevation (in meters)
- State of Charge (SOC) (in percentage)
- Battery voltage (in volts)
- Battery current (in amperes)
It is essential to ensure you understand what each variable represents so you can process and analyze it correctly.
Step 3: Data Processing and Conversion
Once the data is loaded, the next step is to process it. This typically involves converting units to a standardized format (e.g., converting speed from miles per hour to kilometers per hour, or converting voltage to kilovolts). In MATLAB, simple arithmetic operations can be applied to entire columns of data.
For example, if the speed is given in miles per hour (mph) and the task requires converting it to kilometers per hour (km/h), you would multiply the speed by 1.60934 (since 1 mph = 1.60934 km/h):
% Convert speed from miles per hour to kilometers per hour
data.Speed = data.Speed * 1.60934;
This operation applies the conversion factor to every value in the Speed column of the data.
Similarly, if the assignment requires converting temperatures from Fahrenheit to Celsius, or battery voltages from volts to kilovolts, you can apply the appropriate formulas.
% Convert battery voltage to kilovolts
data.PackVolts = data.PackVolts / 1000; % Convert from V to kV
By converting all the relevant data columns into the appropriate units, you ensure that your subsequent calculations are accurate and meaningful.
Step 4: Perform Calculations and Analysis
MATLAB assignments often require you to perform some calculations. These could involve basic mathematical operations, such as multiplying two variables, or more complex tasks like calculating power consumption from voltage and current. For example, one common task in electric vehicle assignments is calculating the battery power using the formula:
Where:
- P is the battery power in kilowatts (kW),
- U is the battery voltage in volts,
- I is the battery current in amperes.
In MATLAB, you can compute the battery power as follows:
% Calculate battery power in kW
P = (data.PackVolts .* data.PackAmps) / 1000;
This calculation involves multiplying the battery voltage and current for each data point and then dividing by 1000 to convert the result to kilowatts. Once you’ve calculated the power, you can use it in subsequent plots or analyses.
For example, if you also need to calculate motor power, auxiliary power, and air conditioning power from the dataset, you could perform similar operations based on the data provided and unit conversion guidelines.
Step 5: Visualization and Plotting
A critical component of most MATLAB assignments is presenting your results visually. MATLAB’s plotting functions are versatile and allow you to create a wide range of plots. One common requirement is to plot multiple variables against time.
For example, to plot several variables (such as speed, battery voltage, and SOC) on subplots, you can use the subplot() function:
% Create subplots for each variable
subplot(2,3,1); plot(data.Time, data.Speed); title('Speed vs Time');
subplot(2,3,2); plot(data.Time, data.SOC); title('SOC vs Time');
subplot(2,3,3); plot(data.Time, data.PackVolts); title('Voltage vs Time');
subplot(2,3,4); plot(data.Time, data.PackAmps); title('Current vs Time');
subplot(2,3,5); plot(data.Time, data.Elv); title('Elevation vs Time');
subplot(2,3,6); plot(data.Time, data.Gear); title('Gear vs Time');
This code creates a 2x3 grid of subplots, where each subplot corresponds to a different variable plotted against time. The title() function gives each subplot a title, and xlabel() and ylabel() can be added for axis labels.
Another common task is to plot multiple variables on the same graph, using different line styles for each one. For example, if you want to plot motor power, auxiliary power, air conditioning power, and battery power on the same figure, you can use:
% Plot power components on the same graph
figure;
hold on;
plot(data.Time, MotorPwr, 'r-'); % Motor power
plot(data.Time, AuxPwr, 'b--'); % Auxiliary power
plot(data.Time, ACPwr, 'g:'); % A/C power
plot(data.Time, P, 'k-.'); % Battery power
hold off;
% Add labels and legend
xlabel('Time (s)');
ylabel('Power (kW)');
legend('Motor Power', 'Auxiliary Power', 'A/C Power', 'Battery Power');
title('Power Distribution Over Time');
grid on;
This code creates a single plot where each power component is represented by a different line style. The legend() function adds a legend to distinguish between the different powers, and the grid on command adds a grid to the figure for easier reading.
Step 6: Summary Statistics
In many assignments, you will need to calculate summary statistics, such as the minimum, maximum, and average of certain variables. For example, if you need to analyze the temperature from multiple sensors, you can use the min(), max(), and mean() functions in MATLAB.
% Calculate minimum, maximum, and average temperature
minT1 = min(data.PackT1);
maxT1 = max(data.PackT1);
avgT1 = mean(data.PackT1);
Once you've computed the summary statistics, you can visualize the results using bar charts or 3D plots. For example, to plot the minimum, maximum, and average temperatures for each sensor, you could use:
% Plot temperature data in a 3D bar chart
figure;
bar3([minT1, maxT1, avgT1; ...]);
xlabel('Sensor');
ylabel('Metric');
zlabel('Temperature (°C)');
title('Battery Pack Temperature');
This code generates a 3D bar chart that displays the temperature statistics for each sensor.
Step 7: Documentation and Code Quality
High-quality MATLAB assignments not only require correct calculations and plots but also well-documented code. Proper documentation helps others (and yourself) understand the logic behind your solution.
- Commenting: Use comments to explain the purpose of each section of your code and describe any complex calculations.
- Indentation: Proper indentation makes your code more readable and ensures that loops and conditional statements are clearly defined.
% Calculate battery power using voltage and current
batteryPower = (data.PackVolts .* data.PackAmps) / 1000; % Power in kW
for i = 1:length(data)
% Process each row of data
power(i) = (data.PackVolts(i) * data.PackAmps(i)) / 1000;
end
By following these coding standards, you will improve the quality of your MATLAB assignment and make it easier to understand and debug.
Conclusion
MATLAB is a powerful tool for data analysis, computation, and visualization, and with the right approach, solving assignments involving data like that of an electric vehicle becomes manageable and even enjoyable. The key steps involve understanding the problem, processing the data, performing calculations, visualizing the results, and ensuring your code is well-documented. By following this guide, you can improve your skills in MATLAB and confidently complete assignments that require data analysis and visualization. If you ever feel stuck or need expert help with MATLAB assignment, don’t hesitate to reach out for professional assistance. There are various services available where experts can help you understand the concepts better and guide you through the process, so you can complete your assignment with ease.