Engineering Design Optimization with MATLAB for Pressure Vessel Design
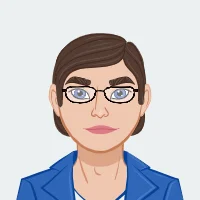
In the world of engineering, design optimization is a crucial process that allows engineers to find the best possible solutions under given constraints. One common application is the design of pressure vessels, essential components in various engineering sectors. This comprehensive guide will walk you through the process of solving similar optimization problems using MATLAB, providing a structured approach to mastering this powerful tool.
Understanding the Fundamentals of Engineering Design Optimization
Engineering design optimization involves formulating a problem to find the optimal solution that meets specific requirements and constraints. The process can be broken down into several key steps:
1. Identify Design Variables:
- Determine the parameters that can be controlled or modified. For instance, in the design of a pressure vessel, the internal radius (ri), length (L), and thickness (t) are typical design variables.
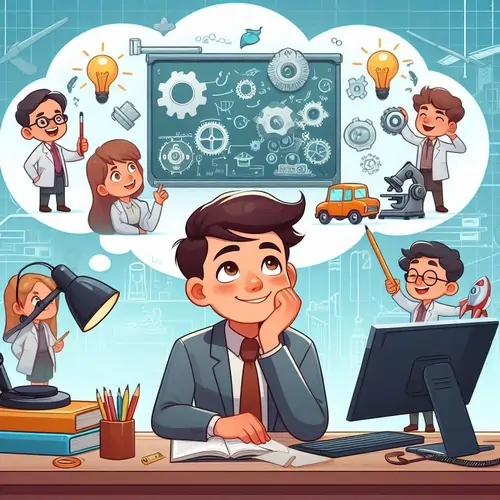
2. Identify Design Qualities:
- These are the qualities or objectives that need to be optimized. Examples include minimizing the weight of the pressure vessel, reducing costs, or maximizing safety.
3. Select Optimization Objective:
- Choose a primary goal for the optimization. For example, you might aim to minimize the vessel’s mass while ensuring it can withstand internal pressure.
4. State All Constraints:
- List all limitations or requirements, such as allowable stress, internal volume, overall size, and dry mass. These constraints ensure the design meets necessary safety and performance standards.
5. Handle Equality Constraints:
- Incorporate any equality constraints into the optimization formulation. An example would be ensuring that the internal volume of the vessel equals the desired capacity.
Step-by-Step Guide to Coding the Optimization Problem in MATLAB
To solve an engineering design optimization problem, you need to structure your MATLAB assignment effectively. Here's how you can do it:
Structuring the Code:
- Organize your code into multiple files for clarity and reusability. Typically, you will have a main script, function files, and possibly a GUI file.
Defining the Optimization Function:
- Create a function that computes the objective and constraints. This function will be used by MATLAB’s optimization solvers.
Here’s an example function for optimizing a pressure vessel:
function [f, constraints] = vesselOptimization(x, P, C, nd, LT_c, H_c)
% x contains the design variables: [ri, L, t]
ri = x(1); L = x(2); t = x(3);
% Calculate derived quantities
Vi = pi * ri^2 * L + 4 * pi * ri^3 / 3;
sigma_allowable = Sy / nd;
sigma_vonMises = sqrt((sigma_t - sigma_r)^2 + (sigma_t - sigma_l)^2 + (sigma_l - sigma_r)^2) / sqrt(2);
% Constraints
constraints = [
sigma_vonMises - sigma_allowable; % Stress constraint
Vi - C; % Volume constraint
LT - LT_c; % Overall length constraint
H - H_c; % Overall height constraint
md - max_mass % Mass constraint
];
% Objective (e.g., minimize mass)
f = Vmat * density;
end
This function takes the design variables and other parameters as inputs and returns the objective function value and constraints. The constraints ensure the design meets all specified requirements.
Developing a Graphical User Interface (GUI) in MATLAB
Creating a user-friendly GUI can enhance the usability of your optimization program. MATLAB provides tools like App Designer and GUIDE to help you build interactive interfaces.
Creating the GUI:
- Use MATLAB’s App Designer or GUIDE to create a user interface for input and output. The GUI should allow users to input necessary parameters and view the results.
GUI Components:
- Inputs: Internal pressure, capacity, factor of safety, constraints on length and height.
- Outputs: Optimal dimensions, material index, type of solution, allowable stress, von Mises stress, material volume, cost index, overall dimensions, and dry mass.
Here’s an example of how you can create a simple GUI:
function runOptimizationApp
fig = uifigure('Name', 'Pressure Vessel Optimization');
% Create input fields
lblPressure = uilabel(fig, 'Position', [10 350 100 22], 'Text', 'Internal Pressure (bar):');
edtPressure = uieditfield(fig, 'numeric', 'Position', [120 350 100 22]);
lblCapacity = uilabel(fig, 'Position', [10 320 100 22], 'Text', 'Capacity (litres):');
edtCapacity = uieditfield(fig, 'numeric', 'Position', [120 320 100 22]);
lblFoS = uilabel(fig, 'Position', [10 290 100 22], 'Text', 'Factor of Safety:');
edtFoS = uieditfield(fig, 'numeric', 'Position', [120 290 100 22]);
lblLT_c = uilabel(fig, 'Position', [10 260 100 22], 'Text', 'Max Length (m):');
edtLT_c = uieditfield(fig, 'numeric', 'Position', [120 260 100 22]);
lblH_c = uilabel(fig, 'Position', [10 230 100 22], 'Text', 'Max Height (m):');
edtH_c = uieditfield(fig, 'numeric', 'Position', [120 230 100 22]);
% Create run button
btnRun = uibutton(fig, 'Position', [10 200 100 22], 'Text', 'Run Optimization', ...
'ButtonPushedFcn', @(btn, event) runOptimization(edtPressure.Value, edtCapacity.Value, edtFoS.Value, edtLT_c.Value, edtH_c.Value));
% Create output display
txaOutput = uitextarea(fig, 'Position', [10 10 380 180], 'Editable', 'off');
% Callback function for the run button
function runOptimization(P, C, nd, LT_c, H_c)
% Call the optimization solver
options = optimoptions('fmincon', 'Display', 'iter');
[x_opt, fval] = fmincon(@(x) vesselOptimization(x, P, C, nd, LT_c, H_c), x0, [], [], [], [], lb, ub, [], options);
% Display the results
txaOutput.Value = sprintf('Optimal dimensions: ri = %.2f, L = %.2f, t = %.2f\n', x_opt(1), x_opt(2), x_opt(3));
end
end
This code creates a simple GUI with input fields for pressure, capacity, factor of safety, and constraints. It also includes a button to run the optimization and a text area to display the results.
Using MAT-Files for Data Storage
MAT-files are a convenient way to store and load data in MATLAB. For example, you can save material properties in a MAT-file for easy access during optimization.
Saving Data:
- Save data, such as material properties, in a MAT-file for easy access.
% Saving data
mat.number = [1, 2, 3, ...];
mat.sy = [170, 280, 180, ...];
mat.t = {[2, 4, 6, 8, 10, 20, 30], [2, 3, 4, ...], ...};
mat.cf = [1, 1.37, 1.1, ...];
save('MaterialFile.mat', 'mat');
Loading Data:
- Load data from a MAT-file when needed.
load('MaterialFile.mat');
By using MAT-files, you can efficiently manage large datasets and ensure your optimization program has quick access to necessary information.
Structuring the Program for Clarity and Reusability
Properly structuring your program is crucial for clarity and reusability. Here are some tips for organizing your MATLAB code:
Organize Files:
- Main script: This file runs the optimization and displays results.
- Function files: These files contain functions for calculating objectives and constraints.
- GUI file: This file contains the code for the graphical user interface.
Example Program Structure
Main Script:
% Main script for running the optimization
clear; clc;
% Load material data
load('MaterialFile.mat');
% Define initial guess and bounds
x0 = [0.5, 1.0, 0.01];
lb = [0.1, 0.1, 0.001];
ub = [2.0, 5.0, 0.1];
% Define parameters
P = 100; % Pressure in bars
C = 1000; % Capacity in litres
nd = 2; % Factor of safety
LT_c = 10; % Max length in meters
H_c = 3; % Max height in meters
% Run optimization
options = optimoptions('fmincon', 'Display', 'iter');
[x_opt, fval] = fmincon(@(x) vesselOptimization(x, P, C, nd, LT_c, H_c), x0, [], [], [], [], lb, ub, [], options);
% Display results
disp('Optimal dimensions:');
disp(['ri = ', num2str(x_opt(1)), ' m']);
disp(['L = ', num2str(x_opt(2)), ' m']);
disp(['t = ', num2str(x_opt(3)), ' m']);
Function File (vesselOptimization.m):
function [f, constraints] = vesselOptimization(x, P, C, nd, LT_c, H_c)
% x contains the design variables: [ri, L, t]
ri = x(1); L = x(2); t = x(3);
% Calculate derived quantities
Vi = pi * ri^2 * L + 4 * pi * ri^3 / 3;
sigma_allowable = Sy / nd;
sigma_vonMises = sqrt((sigma_t - sigma_r)^2 + (sigma_t - sigma_l)^2 + (sigma_l - sigma_r)^2) / sqrt(2);
% Constraints
constraints = [
sigma_vonMises - sigma_allowable; % Stress constraint
Vi - C; % Volume constraint
LT - LT_c; % Overall length constraint
H - H_c; % Overall height constraint
md - max_mass % Mass constraint
];
% Objective (e.g., minimize mass)
f = Vmat * density;
end
GUI File (runOptimizationApp.m):
function runOptimizationApp
fig = uifigure('Name', 'Pressure Vessel Optimization');
% Create input fields
lblPressure = uilabel(fig, 'Position', [10 350 100 22], 'Text', 'Internal Pressure (bar):');
edtPressure = uieditfield(fig, 'numeric', 'Position', [120 350 100 22]);
lblCapacity = uilabel(fig, 'Position', [10 320 100 22], 'Text', 'Capacity (litres):');
edtCapacity = uieditfield(fig, 'numeric', 'Position', [120 320 100 22]);
lblFoS = uilabel(fig, 'Position', [10 290 100 22], 'Text', 'Factor of Safety:');
edtFoS = uieditfield(fig, 'numeric', 'Position', [120 290 100 22]);
lblLT_c = uilabel(fig, 'Position', [10 260 100 22], 'Text', 'Max Length (m):');
edtLT_c = uieditfield(fig, 'numeric', 'Position', [120 260 100 22]);
lblH_c = uilabel(fig, 'Position', [10 230 100 22], 'Text', 'Max Height (m):');
edtH_c = uieditfield(fig, 'numeric', 'Position', [120 230 100 22]);
% Create run button
btnRun = uibutton(fig, 'Position', [10 200 100 22], 'Text', 'Run Optimization', ...
'ButtonPushedFcn', @(btn, event) runOptimization(edtPressure.Value, edtCapacity.Value, edtFoS.Value, edtLT_c.Value, edtH_c.Value));
% Create output display
txaOutput = uitextarea(fig, 'Position', [10 10 380 180], 'Editable', 'off');
% Callback function for the run button
function runOptimization(P, C, nd, LT_c, H_c)
% Call the optimization solver
options = optimoptions('fmincon', 'Display', 'iter');
[x_opt, fval] = fmincon(@(x) vesselOptimization(x, P, C, nd, LT_c, H_c), x0, [], [], [], [], lb, ub, [], options);
% Display the results
txaOutput.Value = sprintf('Optimal dimensions: ri = %.2f, L = %.2f, t = %.2f\n', x_opt(1), x_opt(2), x_opt(3));
end
end
Conclusion
By following these guidelines, you can effectively solve engineering design optimization problems in MATLAB. From understanding the fundamentals to developing a user-friendly GUI, this comprehensive approach ensures you can tackle even the most complex optimization challenges. Remember to validate your code and results thoroughly to ensure accuracy and reliability. Good luck with your matlab assignments!