Designing a State Machine and Real-Time Function for a Biphasic Pulse Generator in MATLAB
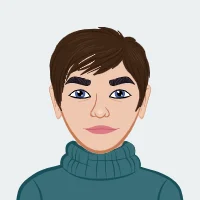
MATLAB assignments can often seem intimidating, especially when they involve intricate tasks like designing state machines and real-time functions. However, with a structured approach and the right strategies, you can navigate through these challenges successfully. In this blog, we’ll guide you on how to approach and solve your MATLAB assignment, such as designing a state machine and corresponding real-time function for a 2-channel charge-balanced cathodic-first biphasic pulse generator. While we’ll use this example as a reference, the strategies shared will be applicable to a wide range of similar assignments.
1. Thoroughly Understand the Problem Statement
The first and most crucial step in tackling any MATLAB assignment is to fully comprehend the problem statement. Jumping into coding or diagramming without a solid understanding of the assignment can lead to mistakes and wasted time. Let’s break down what this process looks like:
Analyze the Requirements
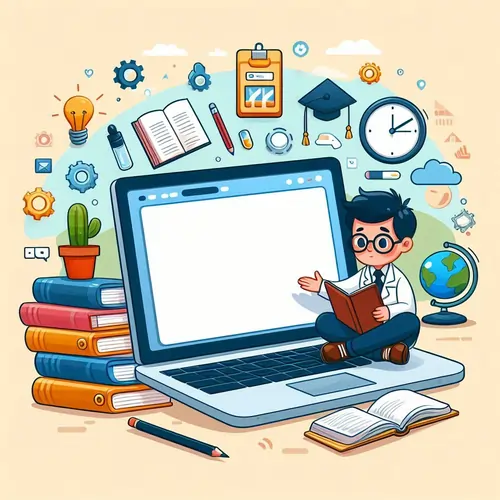
Before you start working on the assignment, read the problem statement multiple times. Identify the key components and requirements:
- State Machine Design: Determine the states involved in the system. For example, in a biphasic pulse generator, states might include "Idle," "Pulse Initiation," "Pulse Continuation," and "Charge Balancing."
- Real-Time Function: Understand how the function will operate in real-time. Consider the constraints like the real-time interrupt interval (10 µs in this case) and the fact that only one channel can be active at a time.
Highlight Key Information
As you read through the problem, highlight or underline key variables and constraints. For instance, in the pulse generator assignment, important variables include AMPA, AMPB (amplitude), PWA, PWB (pulse width), IPA, IPB (inner phase timing), and PRA, PRB (pulse rate). Understanding how these variables interact will be essential when designing your solution.
Create a Mental Model
Visualize how the system is supposed to work. Try to mentally map out the states and transitions before putting pen to paper or fingers to the keyboard. This mental model will serve as a guide when you start designing the state machine and coding the MATLAB function.
Tip: Don’t rush through this phase. Spend adequate time ensuring that you fully understand what is expected. This solid foundation will make the subsequent steps much smoother.
2. Break Down the Assignment into Manageable Tasks
Once you have a clear understanding of the problem, the next step is to break it down into smaller, more manageable tasks. This approach helps in preventing overwhelm and allows you to tackle each part of the assignment systematically.
Task 1: Identify States and Transitions
Start by identifying all possible states of the system. In the context of a state machine, a state represents a specific condition or situation during the operation of the system. For example:
- Idle State: The system is waiting for a trigger to start the pulse.
- Pulse Initiation State: The system starts generating a pulse based on the input parameters.
- Charge Balancing State: Ensures that the pulses are charge balanced, maintaining the integrity of the system.
After identifying the states, determine the transitions between them. A transition is a movement from one state to another based on specific conditions. For example, a transition from "Idle" to "Pulse Initiation" might occur when the pulse rate (PR) is greater than zero.
Task 2: Define Input and Output Variables
Identify and clearly define the input and output variables for your system. For the 2-channel pulse generator assignment, the key input variables include:
- AMPA and AMPB: The amplitude of the cathodic and anodic pulses.
- PWA and PWB: The pulse width for each channel.
- IPA and IPB: The timing between pulses.
- PRA and PRB: The pulse rate.
The output variables would typically be the current values for each channel at any given time.
Task 3: Develop a State Diagram
With your states and transitions identified, the next step is to visually represent them in a state diagram. A state diagram helps you and others understand the flow of the system.
- Draw Each State: Use circles or boxes to represent each state. Ensure that each state is distinct and labeled clearly.
- Draw Transitions: Use arrows to show how the system moves from one state to another. Label these arrows with the conditions that trigger the transition.
- Consider Time Increments: Since this system operates in real-time, include time increments in your state diagram. For instance, if the RTI interval is 10 µs, ensure that your transitions account for this timing.
Task 4: Write and Test the MATLAB Function
Once your state diagram is complete, the next step is to translate it into MATLAB code. Start by writing the core function that will control the state transitions and output the necessary values.
- Pseudo-Code Approach: Before you start coding, consider writing out your logic in pseudo-code. This step allows you to organize your thoughts and outline the function’s logic without worrying about syntax.
- Translate into MATLAB Code: With your pseudo-code as a guide, begin coding the function in MATLAB. Ensure that your code correctly implements the states and transitions you’ve mapped out in the diagram.
- Testing: As you write the function, test it frequently. Use MATLAB’s built-in tools to run simulations and check that the output behaves as expected.
Tip: Keep your code modular. Break down large chunks of logic into smaller functions if necessary. This not only makes your code easier to understand but also simplifies debugging.
3. Design the State Machine Diagram
A well-designed state machine diagram is essential for understanding how your system operates. It provides a clear and concise visual representation of the states and transitions that your system will undergo during its operation.
Drawing the Diagram
Here’s a step-by-step approach to creating an effective state machine diagram:
- Step 1: Draw Each State: Start by representing each state with a circle or box. Label each state clearly with a descriptive name. For example, you might label a state "Idle" if the system is waiting for input, or "Pulse Initiation" if the system is starting to generate a pulse.
- Step 2: Define Transitions: Next, draw arrows between the states to show how the system transitions from one state to another. Each arrow should be labeled with the condition that triggers the transition. For instance, an arrow from "Idle" to "Pulse Initiation" might be labeled with the condition "PR > 0."
- Step 3: Include Time Increments: Since the system operates in real-time, include timing information in your diagram. For example, if a transition occurs after 10 µs, make sure to note that in the diagram.
- Step 4: Review and Revise: Once your diagram is complete, review it carefully. Ensure that it accurately represents the system and that all states and transitions are accounted for.
Software Tools for Diagramming
You can use various tools to create your state machine diagram:
- Hand-Drawn Diagrams: If you prefer a more manual approach, you can draw the diagram by hand. This method allows for quick sketching and revision.
- Software Tools: If you prefer digital tools, software like Microsoft PowerPoint, Lucidchart, or even MATLAB’s built-in tools can be used to create clean, professional-looking diagrams.
Tip: Choose the tool that you’re most comfortable with. The goal is to create a clear and accurate representation of the system, so use whatever method helps you achieve that.
4. Simulate the Real-Time Function in MATLAB
Simulation is a critical part of any complex MATLAB assignment. It allows you to test your system in a controlled environment and ensures that it behaves as expected.
Using Provided Code as a Starting Point
Many assignments come with a starter code or template that you can build upon. In the pulse generator example, a MATLAB script is provided that initializes the system and runs a hardware emulator loop. Here’s how to approach this step:
- Step 1: Run the Provided Script: Start by running the script without making any changes. This will help you understand how the system operates and what outputs it generates.
- Step 2: Analyze the Output: Observe the output generated by the script. Note any patterns or behaviors that are relevant to your assignment.
- Step 3: Implement Your State Machine: Once you’re familiar with the script, replace the placeholder function with your own state machine implementation. Ensure that your function handles all possible states and transitions correctly.
Testing and Debugging
Testing is a crucial step in ensuring that your function operates correctly. Here’s how to approach testing and debugging:
- Test for Edge Cases: Run multiple tests with different input values to ensure that your function behaves as expected in all scenarios. Pay particular attention to edge cases, such as when pulse parameters change mid-operation.
- Use Breakpoints and Debugging Tools: MATLAB’s debugging tools, such as breakpoints and the command window, can be invaluable in identifying and fixing issues in your code. Use these tools to step through your code and observe how it behaves at each stage.
Importance of Semaphore in Real-Time Functions
In real-time systems, proper handling of resources is critical. In the pulse generator assignment, this means correctly implementing a semaphore to prevent pulse clashing. A semaphore is a variable used to control access to a common resource in a concurrent system, ensuring that only one process uses the resource at a time.
Tip: If you notice pulse clashing or other unexpected behaviors during testing, revisit your semaphore implementation. Ensure that it correctly manages access to the single current source, preventing both channels from outputting pulses simultaneously.
5. Document Your Work Effectively
Clear documentation is just as important as the code itself. In MATLAB assignments, proper documentation helps others understand your logic and makes it easier to revisit your work in the future.
Writing Comments in Your Code
Comments in your code provide explanations and clarify your logic. Here’s how to effectively use comments:
- Explain Complex Logic: For any complex or non-obvious code, include a comment explaining what the code does and why it’s necessary. For example, if you use a specific formula to calculate pulse timings, explain the logic behind it.
- Document State Transitions: In your state machine function, include comments that describe each state and the conditions for transitions.
- Label Important Sections: Use comments to label important sections of your code, such as initialization, main loop, and termination.
Creating a Report
For complex assignments, you may be required to submit a report along with your code. This report should provide a detailed explanation of your approach, including:
- Overview: A brief summary of the problem statement and your solution.
- State Machine Design: A description of your state machine, including the states, transitions, and timing considerations.
- MATLAB Function: An explanation of your MATLAB function, including key sections of the code and how they implement the state machine.
- Simulation Results: A summary of your simulation results, including any graphs or output data that demonstrate the correct operation of your system.
Conclusion
Designing a state machine and corresponding real-time function for a complex system like a biphasic pulse generator can be challenging. However, by thoroughly understanding the problem, breaking it down into manageable tasks, designing an effective state machine diagram, and thoroughly testing your MATLAB function, you can successfully complete your assignment. Remember, clear documentation and consistent testing are key to ensuring your solution is both correct and easy to understand.
Whether you're tackling a state machine design or taking assistance with electrical engineering assignment, these strategies will help you navigate the challenges with confidence and precision.